Understanding JavaScript Promise Methods: A Straightforward Guide
JavaScript, the backbone of modern web development, empowers developers to create dynamic and interactive web applications. One key feature that enhances the efficiency of handling asynchronous operations in JavaScript is Promises. In this article, we’ll take a closer look at JavaScript Promise methods in a straightforward manner.
Table of Contents
What are Promises?

How Promises Work
-
Creation: To create a Promise, you use the
Promise
constructor. It takes a function as an argument, commonly referred to as the “executor.” This executor function receives two function arguments:resolve
andreject
. Inside the executor, you perform your asynchronous operation and callresolve
upon success (passing the result) orreject
upon failure (passing an error or reason).const myPromise = new Promise((resolve, reject) => { // Simulating an asynchronous operation (e.g., fetching data, timer) setTimeout(() => { const operationSuccessful = true; // Change to false to test rejection if (operationSuccessful) { // If successful, call resolve with the result resolve('Operation completed successfully!'); } else { // If failed, call reject with an error or reason reject(new Error('Operation failed!')); // It's good practice to reject with an Error object } }, 1000); // Simulating a 1-second delay });
-
States: A Promise can be in one of three states:
- Pending: The initial state; the asynchronous operation has not yet completed.
- Fulfilled: The operation completed successfully, and the Promise now has a resolved value.
- Rejected: The operation failed, and the Promise has a reason for the failure (usually an error object).
-
Consuming Promises: You use the
.then()
method to schedule code to run when the Promise is fulfilled. You use the.catch()
method to handle errors when the Promise is rejected. You can also use.finally()
to execute code regardless of whether the promise was fulfilled or rejected.myPromise .then((result) => { // This function runs if the promise is resolved console.log('Success:', result); }) .catch((error) => { // This function runs if the promise is rejected console.error('Error:', error); }) .finally(() => { // This function runs always, after fulfillment or rejection console.log('Promise settled (either fulfilled or rejected).'); });
Promise Methods
JavaScript provides several static methods on the Promise
object itself to work with multiple promises or create promises in specific states.
1. Promise.all()
Used when you have multiple promises and need to wait until all of them have successfully fulfilled. If any promise in the input array rejects, the entire Promise.all()
immediately rejects with the reason of the first rejected promise.
- Parameters: An iterable (like an Array) of promises.
- Return Value: A new promise that:
- Resolves with an array containing the fulfilled values of the input promises (in the same order as the input array) once all input promises have fulfilled.
- Rejects with the reason of the first promise that rejects.
const promise1 = Promise.resolve(1);
const promise2 = new Promise(resolve => setTimeout(() => resolve(2), 50)); // Simulate async
const promise3 = Promise.resolve(3);
// Example 1: All resolve
Promise.all([promise1, promise2, promise3])
.then(values => {
console.log('Promise.all resolved:', values); // Output: Promise.all resolved: [1, 2, 3]
})
.catch(error => {
// This won't run in this case
console.error('Promise.all rejected:', error);
});
// Example 2: One rejects
const promise4 = Promise.reject(new Error('Something went wrong'));
Promise.all([promise1, promise4, promise3])
.then(values => {
// This won't run
console.log('Promise.all resolved:', values);
})
.catch(error => {
console.error('Promise.all rejected:', error.message); // Output: Promise.all rejected: Something went wrong
});
2. Promise.resolve()
A shortcut method that returns a Promise
object that is already resolved with the given value. Useful for converting a non-promise value into a promise or starting a promise chain with an initial value.
- Parameters: A value (or another promise) to fulfill the new promise with.
- Return Value: A promise that is resolved with the given value.
const alreadyResolvedValue = 'Resolved value';
const resolvedPromise = Promise.resolve(alreadyResolvedValue);
resolvedPromise.then(value => {
console.log(value); // Output: Resolved value
});
// If you pass a promise, it returns that same promise
const p1 = new Promise(res => setTimeout(() => res("p1 resolved"), 100));
const p2 = Promise.resolve(p1);
console.log(p1 === p2); // Output: true
3. Promise.reject()
Similar to Promise.resolve()
, but returns a Promise
object that is already rejected with the given reason (usually an error object).
- Parameters: A reason (preferably an
Error
object) for the rejection. - Return Value: A promise that is rejected with the specified reason.
const reason = new Error('Custom rejection reason');
const rejectedPromise = Promise.reject(reason);
rejectedPromise.catch(error => {
// Remember to always add .catch() to handle rejected promises
console.error(error.message); // Output: Custom rejection reason
});
4. Promise.race()
Takes an iterable of promises and returns a new promise that settles (either fulfills or rejects) as soon as the first promise in the iterable settles.
- Parameters: An iterable (like an Array) of promises.
- Return Value: A new promise that mirrors the state (fulfillment or rejection) of the first promise in the iterable to settle.
const promiseA = new Promise(resolve => setTimeout(() => resolve('A wins!'), 100));
const promiseB = new Promise((resolve, reject) => setTimeout(() => reject(new Error('B rejects first!')), 50));
const promiseC = new Promise(resolve => setTimeout(() => resolve('C is too slow'), 200));
Promise.race([promiseA, promiseB, promiseC])
.then(result => {
// This won't run because promiseB rejects first
console.log('Promise.race fulfilled:', result);
})
.catch(error => {
console.error('Promise.race rejected:', error.message); // Output: Promise.race rejected: B rejects first!
});
// Another example where one fulfills first
const promiseX = new Promise(resolve => setTimeout(() => resolve('X finishes first!'), 60));
Promise.race([promiseX, promiseB, promiseC])
.then(result => {
console.log('Promise.race fulfilled:', result); // Output: Promise.race fulfilled: X finishes first!
})
.catch(error => {
// This won't run
console.error('Promise.race rejected:', error.message);
});
5. More Promise Methods
The methods explained above (.all()
, .resolve()
, .reject()
, .race()
) are fundamental and frequently used. However, the Promise API has evolved, offering more specialized methods:
Promise.allSettled()
: Waits for all input promises to settle (either fulfill or reject) and returns a promise that resolves with an array of objects describing the outcome of each input promise. Useful when you need to know the result of every promise, regardless of success or failure.Promise.any()
: Takes an iterable of promises and returns a promise that fulfills as soon as any of the input promises fulfills. If all input promises reject, it rejects with anAggregateError
. Useful when you only need one successful outcome from multiple sources.Promise.prototype.catch()
: (Instance method) Appends a rejection handler callback to the promise, and returns a new promise resolving to the return value of the callback if it is called, or to its original fulfillment value if the promise is instead fulfilled.Promise.prototype.finally()
: (Instance method) Appends a handler to the promise, and returns a new promise that is resolved when the original promise is resolved. The handler is called when the promise is settled, whether fulfilled or rejected.Promise.prototype.then()
: (Instance method) Appends fulfillment and rejection handlers to the promise, and returns a new promise resolving to the return value of the called handler, or to its original settled value if the promise was not handled.Promise.withResolvers()
: (Static method - newer addition) Returns an object containing a newPromise
object and two functions,resolve
andreject
, linked to the promise. Allows exposing the resolve/reject capabilities outside the promise executor scope.
All of these methods are explained in more detail on the MDN Web Docs for Promise. Check them out to learn exactly how and when to use them.
Conclusion
JavaScript Promise methods provide a powerful and structured way to manage asynchronous operations. By understanding Promise.all()
, Promise.resolve()
, Promise.reject()
, Promise.race()
, and knowing that other specialized methods exist, you can write clearer, more robust, and more maintainable asynchronous JavaScript code. As you encounter different asynchronous scenarios, these methods will become invaluable tools in your development toolkit.
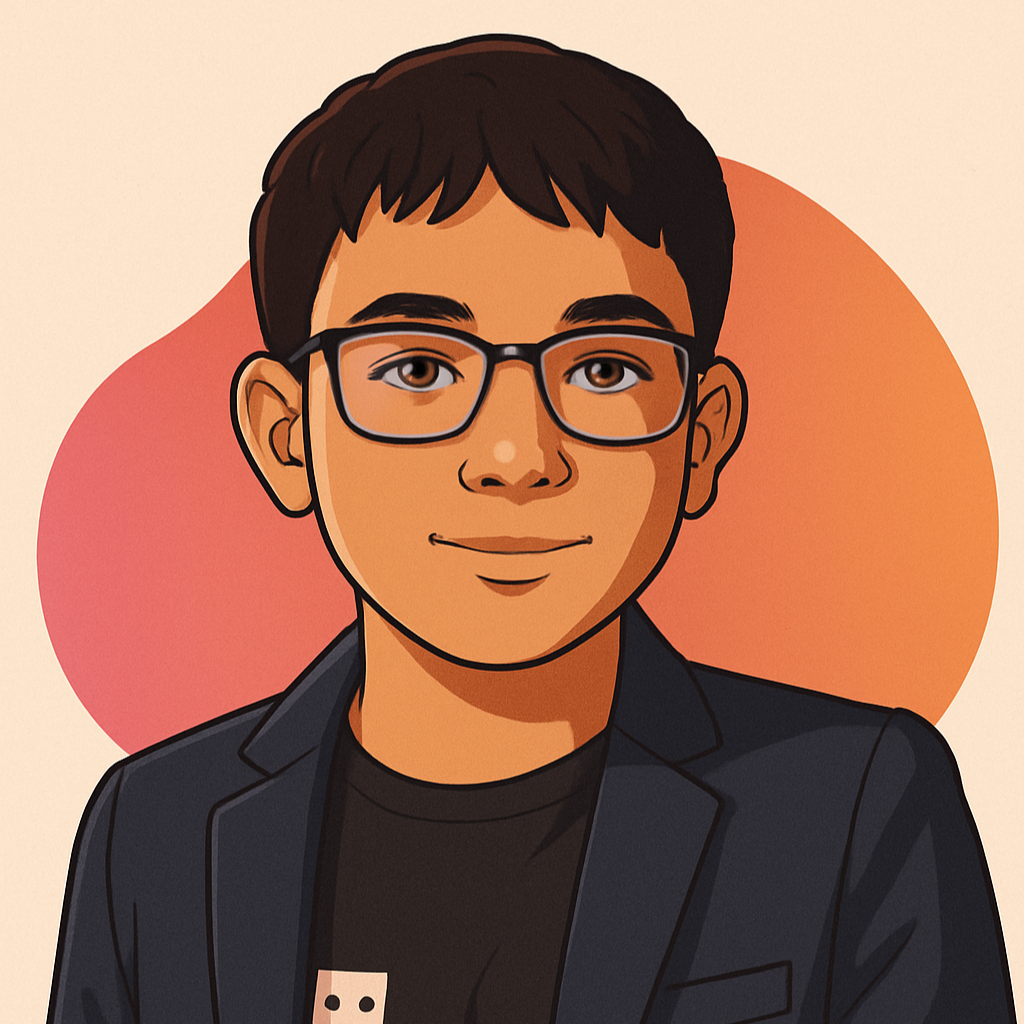
Solo Developer | Python & JS | FastAPI, Django, Next.js, Svelte