Mastering Python F-strings: A Comprehensive Guide to Formatted String Literals
Python, renowned for its readability and simplicity, continually evolves to enhance developer experience. One significant evolution introduced in Python 3.6 is F-strings, a concise, expressive, and highly efficient way to format strings. F-strings, short for “formatted string literals,” offer a more intuitive and readable alternative to older string formatting methods like %-formatting or str.format()
.
This guide aims to provide a comprehensive exploration of F-string formatting in Python, delving into its syntax, capabilities, and practical applications. Whether you’re a Python novice just learning about string manipulation or an experienced developer looking to leverage modern features, understanding F-strings opens up a world of possibilities for cleaner and more efficient code.
In the following sections, we’ll cover the basics of F-strings, demonstrating how they differ from older techniques. We’ll explore variable insertion, embedding expressions within F-strings, and the various powerful formatting options available. Additionally, we’ll delve into real-world use cases, providing practical examples that showcase the versatility and power of F-strings.
By the end of this guide, you’ll not only grasp the fundamentals of F-string formatting but also be equipped with the knowledge to leverage this feature effectively in your Python projects. Let’s embark on a journey into the world of F-strings and discover how they can elevate your string formatting game in Python.
Table of Contents
- 1. Basics of F-strings
- 2. Variable Insertion
- 3. Expressions in F-strings
- 4. Formatting Options
- 5. String Manipulation with F-strings
- 6. F-string and Data Structures
- 7. F-strings and Multiline Strings
- 8. Advanced F-string Techniques
- 9. Best Practices and Tips for F-string Formatting
- Conclusion
1. Basics of F-strings
F-strings, or formatted string literals, were introduced in Python 3.6 (PEP 498) and provide a highly readable and efficient way to embed Python expressions inside string literals.
Syntax: You create an F-string by prefixing the string literal with the letter f
or F
. Inside the string, you enclose Python expressions within curly braces {}
. These expressions are evaluated at runtime and their results are formatted into the string.
language = "Python"
version = 3.10
# Basic F-string
message = f"We are using {language} version {version}."
print(message)
# Output: We are using Python version 3.10.
Compare this to older methods:
- %-formatting (older style):
message_percent = "We are using %s version %.2f." % (language, version) print(message_percent) # Output: We are using Python version 3.10.
str.format()
method (Python 2.6+):message_format = "We are using {} version {}.".format(language, version) print(message_format) # Output: We are using Python version 3.10. message_format_named = "We are using {lang} version {ver}.".format(lang=language, ver=version) print(message_format_named) # Output: We are using Python version 3.10.
While the older methods work, F-strings are generally preferred for their conciseness and readability – the variables/expressions are right where they appear in the output string.
Key points about F-strings:
- Expression Evaluation: Anything inside
{}
is treated as a Python expression and evaluated in the current scope. - Readability: Expressions are embedded directly, making the string’s intent clearer than placeholders or separate arguments.
- Conciseness: Often requires less code than
.format()
or %-formatting. - Performance: F-strings are generally faster than other methods because they are parsed directly into efficient bytecode.
- Version Requirement: Requires Python 3.6 or newer.
2. Variable Insertion
The most common use of F-strings is inserting the values of variables directly into a string.
name = "Alice"
activity = "programming"
hours = 4
# Simple variable insertion
greeting = f"Hello, {name}!"
status = f"{name} spent {hours} hours {activity} today."
print(greeting) # Output: Hello, Alice!
print(status) # Output: Alice spent 4 hours programming today.
Key considerations:
- Automatic Conversion: F-strings automatically call the equivalent of
str()
on the variable’s value before inserting it. You don’t needstr(hours)
.count = 10 item = "apples" message = f"There are {count} {item}." # count is automatically converted to '10' print(message) # Output: There are 10 apples.
- Scope: Any variable accessible in the current scope where the F-string is defined can be used inside the curly braces.
3. Expressions in F-strings
F-strings aren’t limited to just variables; you can embed almost any valid Python expression inside the curly braces {}
. The expression is evaluated, and its result is formatted into the string.
a = 5
b = 10
# Arithmetic operations
calculation = f"The sum of {a} and {b} is {a + b}."
print(calculation) # Output: The sum of 5 and 10 is 15.
# Function calls
name = "Bob"
greeting = f"Hello, {name.upper()}!" # Calling the .upper() string method
print(greeting) # Output: Hello, BOB!
def get_status(user_id):
# In a real app, this might query a database
return "Active" if user_id % 2 == 0 else "Inactive"
user_id = 124
status_message = f"User {user_id}'s status is: {get_status(user_id)}"
print(status_message) # Output: User 124's status is: Active
# Accessing list/dictionary elements
my_list = [10, 20, 30]
my_dict = {"item": "widget", "price": 9.99}
list_access = f"The second element is {my_list[1]}."
dict_access = f"The {my_dict['item']} costs ${my_dict['price']}."
print(list_access) # Output: The second element is 20.
print(dict_access) # Output: The widget costs $9.99.
This allows for powerful inline computations and formatting directly within the string literal.
4. Formatting Options
F-strings support a powerful format specification mini-language inside the curly braces, following the expression, separated by a colon :
. The syntax is {expression:format_specifier}
.
4.1 Precision in Floating-Point Numbers:
Use :.nf
to format a floating-point number to n
decimal places.
pi_value = 3.1415926535
price = 49.95
formatted_pi = f"Pi (3 decimals): {pi_value:.3f}"
formatted_price = f"Price: ${price:.2f}" # Common for currency
print(formatted_pi) # Output: Pi (3 decimals): 3.142 (Note: it rounds)
print(formatted_price) # Output: Price: $49.95
4.2 Integer Formatting:
Format integers, often used for padding with zeros or spaces. Use :nd
for decimal integers, where n
is the minimum width. Use 0
before n
for zero-padding.
quantity = 42
item_id = 7
formatted_quantity = f"Quantity: {quantity:4d}" # Pad with spaces to width 4
formatted_id = f"Item ID: {item_id:03d}" # Pad with zeros to width 3
print(formatted_quantity) # Output: Quantity: 42
print(formatted_id) # Output: Item ID: 007
4.3 Alignment:
Control the alignment within a specified width using <
(left), ^
(center), >
(right). You can also specify a fill character before the alignment operator.
message = "Python"
value = 123
# Align within a width of 10 characters
left_aligned = f"Left: |{message:<10}|"
center_aligned = f"Center: |{message:^10}|"
right_aligned = f"Right: |{message:>10}|"
# With fill character '*'
filled_center = f"Filled: |{message:*^12}|"
filled_right_num = f"Number: |{value:*>8}|" # Right align number, fill with *
print(left_aligned) # Output: Left: |Python |
print(center_aligned) # Output: Center: | Python |
print(right_aligned) # Output: Right: | Python|
print(filled_center) # Output: Filled: |***Python***|
print(filled_right_num) # Output: Number: |*****123|
4.4 String Truncation:
Limit the length of a string using :.n
, where n
is the maximum number of characters to display.
long_text = "This is a very long piece of text that needs shortening."
truncated_text = f"Shortened: {long_text:.20}" # Display only the first 20 characters
print(truncated_text) # Output: Shortened: This is a very long
4.5 Date Formatting:
Use standard datetime
formatting codes after the colon. You need to import the datetime
module first.
from datetime import datetime
current_date = datetime.now()
# Common date/time formats
formatted_date = f"Date: {current_date:%Y-%m-%d}"
formatted_time = f"Time: {current_date:%H:%M:%S}"
formatted_full = f"Full: {current_date:%A, %B %d, %Y %I:%M %p}"
print(formatted_date) # Output: Date: 2023-10-27 (example)
print(formatted_time) # Output: Time: 14:30:55 (example)
print(formatted_full) # Output: Full: Friday, October 27, 2023 02:30 PM (example)
These options provide fine-grained control over the final appearance of your strings.
5. String Manipulation with F-strings
You can perform many string operations directly inside the F-string’s curly braces {}
.
5.1 Concatenation with F-strings:
While F-strings excel at embedding, you can achieve concatenation simply by placing variables/expressions next to each other within the string.
first_name = "John"
last_name = "Doe"
title = "Dr."
# Effectively concatenates the parts
full_name = f"{title} {first_name} {last_name}"
print(full_name) # Output: Dr. John Doe
5.2 String Methods in F-strings:
Call any string method on the variable or expression inside the braces.
text = " extra whitespace "
file_name = "report.TXT"
formatted_text = f"Stripped: '{text.strip()}'" # Remove leading/trailing whitespace
lower_filename = f"Lowercase: {file_name.lower()}"
print(formatted_text) # Output: Stripped: 'extra whitespace'
print(lower_filename) # Output: Lowercase: report.txt
5.3 Format Specification in F-strings (Recap):
As covered in Section 4, format specifiers control padding, alignment, precision etc.
greeting = "Hi"
# Right-aligned, width 10, padded with '-'
formatted_greeting = f"{greeting:->10}"
print(formatted_greeting) # Output: --------Hi
5.4 Case Transformation in F-strings:
Use standard string methods for case changes.
message = "python programming"
book_title = "the lord of the rings"
upper_message = f"Uppercase: {message.upper()}"
title_case_book = f"Title: {book_title.title()}"
print(upper_message) # Output: Uppercase: PYTHON PROGRAMMING
print(title_case_book) # Output: Title: The Lord Of The Rings
5.5 Dynamic String Manipulation (Conditional):
Combine expressions, including conditional (ternary) operators, for dynamic output.
age = 25
score = 85
age_group = f"Age group: {'Child' if age < 18 else 'Adult'}"
result = f"Score: {score} - {'Pass' if score >= 50 else 'Fail'}"
print(age_group) # Output: Age group: Adult
print(result) # Output: Score: 85 - Pass
6. F-string and Data Structures
F-strings make it easy to embed elements from dictionaries, lists, tuples, and other data structures.
6.1 Formatting Dictionaries with F-strings:
Access dictionary values using standard key notation ['key']
inside the braces. Remember to use different quote types for the f-string and the key if needed (e.g., f-string with double quotes, key with single quotes).
person_info = {"name": "Alice", "age": 30, "city": "Wonderland"}
formatted_info = f"{person_info['name']} is {person_info['age']} years old and lives in {person_info['city']}."
# Or using different quotes:
formatted_info_alt = f'{person_info["name"]} is {person_info["age"]}.'
print(formatted_info) # Output: Alice is 30 years old and lives in Wonderland.
print(formatted_info_alt) # Output: Alice is 30.
6.2 Formatting Lists with F-strings:
Access list elements using index notation [index]
inside the braces. You can also use methods like .join()
often combined with generator expressions or list comprehensions for cleaner output of multiple elements.
fruits = ["apple", "banana", "orange"]
scores = [88, 92, 75]
first_fruit = f"The first fruit is {fruits[0]}."
average_score = f"Average score: {sum(scores)/len(scores):.1f}"
# Joining list elements (common pattern)
formatted_fruits = f"Available fruits: {', '.join(fruits)}"
print(first_fruit) # Output: The first fruit is apple.
print(average_score) # Output: Average score: 85.0
print(formatted_fruits) # Output: Available fruits: apple, banana, orange
6.3 Nested Data Structures in F-strings:
Access elements within nested structures by chaining the accessors.
contact = {
"name": "Bob",
"address": {"street": "123 Main St", "city": "Metropolis", "zipcode": "12345"},
"phones": ["555-1111", "555-2222"]
}
formatted_contact = f"{contact['name']}'s city: {contact['address']['city']}"
first_phone = f"Primary phone: {contact['phones'][0]}"
print(formatted_contact) # Output: Bob's city: Metropolis
print(first_phone) # Output: Primary phone: 555-1111
6.4 Using Variables in Data Structure Formatting:
Combine variables defined outside the F-string with data structure access inside the braces.
product = {"name": "Laptop", "price": 1200}
discount_percentage = 15 # Discount as a percentage
# Calculate discounted price inside the f-string
discount_decimal = discount_percentage / 100
formatted_price = f"The {product['name']} costs ${product['price'] * (1 - discount_decimal):.2f} after a {discount_percentage}% discount."
print(formatted_price) # Output: The Laptop costs $1020.00 after a 15% discount.
7. F-strings and Multiline Strings
F-strings work seamlessly with Python’s triple-quoted strings ("""..."""
or '''...'''
) for creating multiline output.
7.1 Basic Multiline F-string:
Use f"""..."""
or f'''...'''
.
name = "Alice"
age = 30
multiline_output = f"""
User Profile:
Name: {name}
Age: {age}
"""
# Note: The indentation inside the triple quotes IS part of the string.
print(multiline_output)
# Output:
# User Profile:
# Name: Alice
# Age: 30
7.2 Expression and Variable Insertion in Multiline F-strings:
Expressions work just like in single-line F-strings.
radius = 5
pi = 3.14159
area_calculation = f"""
Circle Details:
Radius: {radius}
Area: {pi * radius ** 2:.2f}
"""
print(area_calculation)
# Output:
# Circle Details:
# Radius: 5
# Area: 78.54
7.3 Conditional Statements in Multiline F-strings:
Embed ternary operators or other expressions for conditional text.
status = "active"
role = "Admin"
user_message = f"""
User Information:
Status: {status.capitalize()}
Access: {'Full Access Granted' if role == 'Admin' and status == 'active' else 'Limited Access'}
"""
print(user_message)
# Output:
# User Information:
# Status: Active
# Access: Full Access Granted
7.4 Indentation and Formatting in Multiline F-strings:
Whitespace and indentation inside the triple quotes are preserved. This is useful for formatting code snippets or structured text.
function_name = "greet"
parameter = "name"
code_block = f"""
def {function_name}({parameter}):
'''A simple greeting function.'''
print(f"Hello, {{{parameter}}}!")
#{function_name}("World")
"""
# Note the {{}} to escape the inner braces for the print() example
print(code_block)
# Output:
# def greet(name):
# '''A simple greeting function.'''
# print(f"Hello, {name}!")
#
# greet("World")
7.5 Combining Multiline and Single-Line F-strings:
You can concatenate or build up strings using both types as needed.
name = "Bob"
age = 25
city = "Metropolis"
# Build parts and combine
header = f"--- User Record ---"
details = f"""
Name: {name}
Age: {age}
City: {city}
"""
footer = f"--- End Record ---"
full_record = f"{header}\n{details}\n{footer}"
print(full_record)
# Output:
# --- User Record ---
#
# Name: Bob
# Age: 25
# City: Metropolis
#
# --- End Record ---
8. Advanced F-string Techniques
Beyond the basics, F-strings offer more advanced capabilities.
8.1 Dynamic Access using Variables:
Use variables inside the braces to dynamically access elements from collections.
fruit_counts = {"apple": 10, "banana": 5, "orange": 8}
selected_fruit = "banana" # This could come from user input, etc.
# Access the dictionary using the 'selected_fruit' variable
count_of_selected = f"There are {fruit_counts[selected_fruit]} {selected_fruit}s."
print(count_of_selected) # Output: There are 5 bananas.
8.2 Attribute Access in F-strings:
Directly access attributes or call methods of objects within the braces.
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def get_initials(self):
return "".join(part[0].upper() for part in self.name.split())
person_instance = Person(name="Charlie Chaplin", age=88)
formatted_person = f"Person: {person_instance.name} (Age: {person_instance.age})"
initials = f"Initials: {person_instance.get_initials()}" # Calling a method
print(formatted_person) # Output: Person: Charlie Chaplin (Age: 88)
print(initials) # Output: Initials: CC
8.3 Conditional Expressions (Ternary Operator):
Use the concise value_if_true if condition else value_if_false
syntax within braces.
temperature = 15
weather_status = f"The weather is {'cold' if temperature < 10 else 'cool' if temperature < 20 else 'warm'}."
print(weather_status) # Output: The weather is cool.
8.4 String Joining with F-strings:
While .join()
is standard, F-strings can be used within list comprehensions or generator expressions passed to .join()
.
greetings = ["Hello", "Hola", "Bonjour"]
# Format each greeting within the generator expression
joined_greetings = ", ".join(f"'{greeting.upper()}'" for greeting in greetings)
print(joined_greetings) # Output: 'HELLO', 'HOLA', 'BONJOUR'
8.5 Formatted Expressions with F-strings (Recap):
Combine complex expressions with format specifiers.
values = [10.5, 22.1, 15.8]
average = sum(values) / len(values)
# Calculate average and format it
formatted_average = f"Average: {average:.2f}"
print(formatted_average) # Output: Average: 16.13
# Calculate within f-string and format
formatted_calc = f"Direct Average: {(sum(values) / len(values)):.2f}"
print(formatted_calc) # Output: Direct Average: 16.13
8.6 Escaping Characters in F-strings:
To include a literal curly brace {
or }
within an F-string, double it: {{
or }}
.
# Need to show literal braces in the output
text_with_braces = f"To create an f-string, use f'{{expression}}'."
print(text_with_braces) # Output: To create an f-string, use f'{expression}'.
# Example with formatting AND literal braces
value = 10
formatted_with_braces = f"The value is {{{value:03d}}}" # Show {010}
print(formatted_with_braces) # Output: The value is {010}
9. Best Practices and Tips for F-string Formatting
To use F-strings effectively and maintain clean code:
- Consistency: Stick to one style (e.g., always use double quotes for f-strings unless inner quotes require singles).
- Keep Expressions Simple: Avoid overly complex logic or multiple lines of code inside the
{}
. If it gets complicated, calculate the value beforehand and insert the resulting variable.# Less readable: # result = f"Final value: {(lambda x: x*x + 2*x + 1)(some_input):.2f}" # More readable: intermediate_value = (lambda x: x*x + 2*x + 1)(some_input) result = f"Final value: {intermediate_value:.2f}"
- Use Descriptive Variable Names: Clear names make f-strings self-documenting.
f"{user_name}"
is better thanf"{un}"
. - Escape Braces Correctly: Remember
{{
and}}
for literal braces. - Mind Line Length: For very long strings or complex formatting, break them down or use multiline f-strings (
f"""..."""
). Adhere to PEP 8 line length guidelines where practical. - Leverage Format Specifiers: Don’t do manual padding or rounding if a format specifier can do it cleanly (e.g., use
{value:.2f}
instead ofround(value, 2)
inside the f-string if formatting is the main goal). - Combine Wisely: Mix f-strings with regular strings if parts of the string are static.
base_url = "https://example.com/api" user_id = 123 endpoint = f"{base_url}/users/{user_id}" # Combines static and dynamic parts
- Test Incrementally: For complex f-strings, print intermediate parts or test the expressions separately if you encounter issues.
- Use Functions for Complex Logic: Encapsulate complex calculations or formatting logic in functions and call them from within the f-string.
def format_user_status(user): # ... complex logic to determine status string ... return f"Status: {user.status} (Last Seen: {user.last_seen:%Y-%m-%d})" # Usage: status_line = f"User {user.id}: {format_user_status(user)}"
- Version Check: Remember F-strings require Python 3.6+. Ensure compatibility if your code needs to run on older versions (though 3.6 is quite old now).
Conclusion
In the landscape of Python string formatting, F-strings stand out as a modern, concise, highly readable, and efficient tool. Since their introduction in Python 3.6, they have largely become the preferred method for developers constructing strings dynamically. By allowing direct embedding of variables and expressions, coupled with a powerful mini-language for formatting, F-strings significantly enhance code clarity and maintainability compared to older methods.
Throughout this guide, we’ve explored the journey from basic variable insertion and expression evaluation to leveraging advanced formatting options, data structures, multiline strings, and sophisticated techniques. F-strings prove versatile in countless scenarios, including logging, generating reports, constructing database queries, building user interfaces, and much more.
By adhering to best practices—keeping expressions simple, using clear variable names, leveraging format specifiers, and ensuring code readability—you can harness the full power of F-strings effectively.
As you continue your Python development, make F-strings a core part of your toolkit. Their adoption will streamline your string formatting tasks, reduce boilerplate, and contribute to writing more elegant and Pythonic code. Embrace the power and simplicity of F-strings to elevate your programming experience.
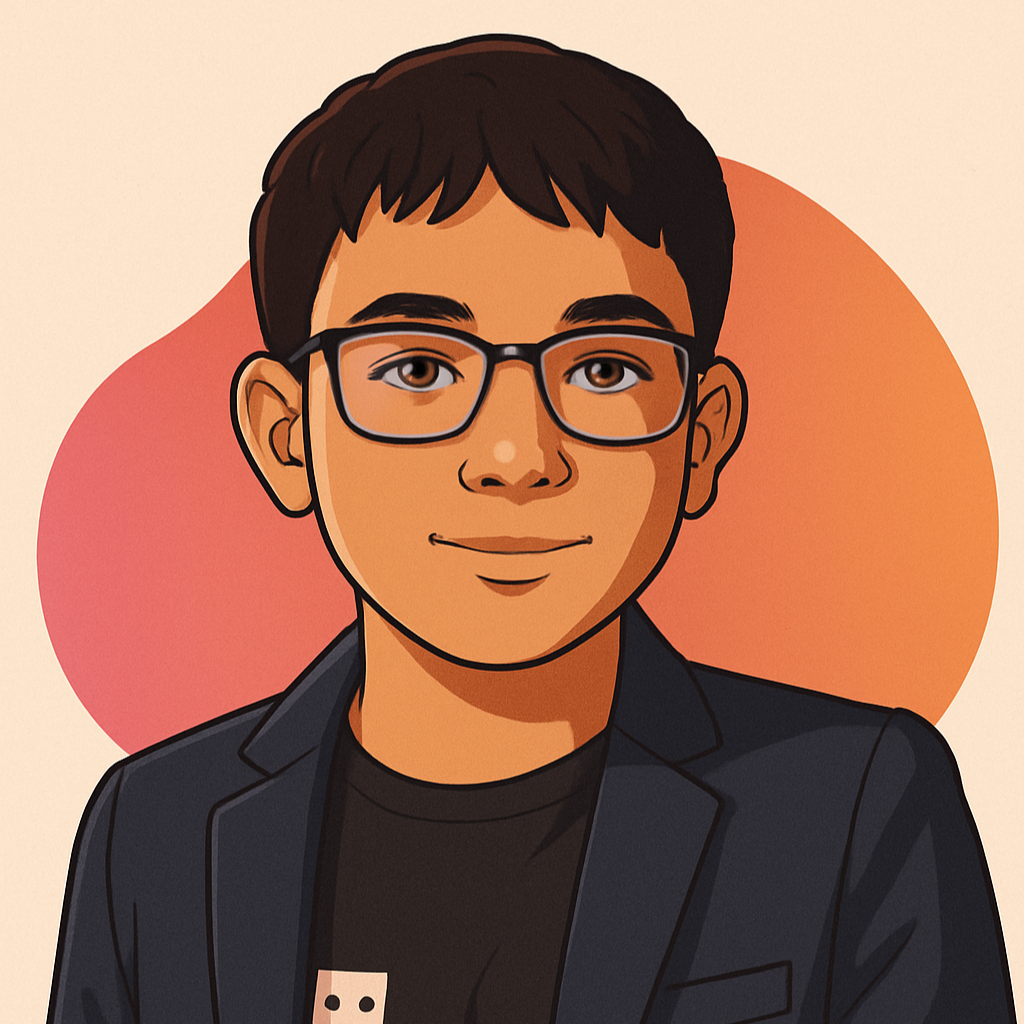
Solo Developer | Python & JS | FastAPI, Django, Next.js, Svelte