We’ve all been there: you write a handy Python script to automate a task, maybe process some files or fetch some data. Initially, you might just change variables directly in the code or use basic input() prompts. But as the script grows or you want others (or your future self!) to use it easily, hardcoding parameters becomes messy and inefficient. This is where Command Line Interface (CLI) tools shine.
A well-designed CLI allows users to interact with your script via the terminal, passing arguments and options to control its behavior without ever touching the source code. Python offers excellent ways to build these interfaces, and two of the most popular choices are the built-in argparse module and the third-party library click.
In this post, we’ll dive into both, showing you how to:
-
Understand the basics of argument parsing.
-
Build a simple CLI tool using
argparse
. -
Build the same tool using click.
-
Compare the two libraries and understand when to use which.
-
Learn best practices for designing user-friendly CLIs.
-
Make your script directly executable.
Let’s get started!
Why Build a CLI Tool?
Before we jump into the code, why bother turning a script into a CLI tool?
-
Automation: CLIs are easily scriptable. You can chain commands together, run them in cron jobs, or integrate them into larger automated workflows.
-
Reusability: Instead of modifying code for different inputs, users can simply pass different arguments.
-
User-Friendliness (for technical users): For developers, sysadmins, and data scientists comfortable with the terminal, CLIs are often faster and more powerful than GUIs.
-
Clarity: Defining explicit arguments and options documents how your script should be used. Both argparse and click automatically generate helpful —help messages.
Argument Parsing Basics
CLIs typically accept two main types of input:
-
Arguments (Positional): These are required values that the script needs to function, and their meaning is determined by their position in the command. Example: cp source.txt destination.txt (source.txt and destination.txt are positional arguments).
-
Options (Flags/Switches): These modify the command’s behavior. They usually have names (e.g., —verbose or -v) and can optionally take values (e.g., —output report.txt or -o report.txt). They are generally order-independent.
Argument parsing libraries help you define these arguments and options, parse them from the command line input (sys.argv), handle errors (like missing arguments or incorrect types), and generate help messages.
Deep Dive: argparse - The Built-in Standard
argparse is part of Python’s standard library, meaning you don’t need to install anything extra. It’s powerful and flexible, though sometimes considered a bit verbose.
Core Concept: You create an ArgumentParser object, add arguments and options to it using add_argument(), and then parse the command-line arguments using parse_args().
Example: A Simple Greeting Tool
Let’s build a tool greet.py
that greets a person, optionally using a specific greeting phrase and optionally making the output uppercase.
# greet_argparse.py
import argparse
def greet(name, greeting="Hello", uppercase=False):
"""Generates a greeting message."""
message = f"{greeting}, {name}!"
if uppercase:
message = message.upper()
print(message)
if __name__ == "__main__":
# 1. Initialize the Parser
parser = argparse.ArgumentParser(
description="A simple program that greets the user."
)
# 2. Add Positional Argument: name
parser.add_argument(
"name",
help="The name of the person to greet."
)
# 3. Add Optional Argument: --greeting / -g
parser.add_argument(
"-g", "--greeting",
default="Hello",
help="The greeting phrase to use (default: Hello)."
)
# 4. Add Optional Argument (Flag): --uppercase / -u
parser.add_argument(
"-u", "--uppercase",
action="store_true", # Makes it a flag (no value needed)
help="Print the greeting in uppercase."
)
# 5. Parse the arguments
args = parser.parse_args()
# 6. Call the core logic
greet(name=args.name, greeting=args.greeting, uppercase=args.uppercase)
How to Run It:
Save this as greet_argparse.py
.
# Basic usage (Positional argument is required)
$ python greet_argparse.py Alice
Hello, Alice!
# Using the --greeting option
$ python greet_argparse.py Bob --greeting "Good morning"
Good morning, Bob!
# Using the short -g option
$ python greet_argparse.py Charlie -g Hi
Hi, Charlie!
# Using the --uppercase flag
$ python greet_argparse.py David --uppercase
HELLO, DAVID!
# Combining options
$ python greet_argparse.py Eve -g Welcome --uppercase
WELCOME, EVE!
# Getting help (automatically generated!)
$ python greet_argparse.py --help
usage: greet_argparse.py [-h] [-g GREETING] [-u] name
A simple program that greets the user.
positional arguments:
name The name of the person to greet.
options:
-h, --help show this help message and exit
-g GREETING, --greeting GREETING
The greeting phrase to use (default: Hello).
-u, --uppercase Print the greeting in uppercase.
argparse
Pros:
-
Built-in: No external dependencies needed.
-
Powerful & Flexible: Handles complex scenarios, custom types, sub-parsers (for commands like git commit, git push), etc.
-
Standard: Widely used and understood in the Python community.
argparse
Cons:
-
Verbose: Defining arguments can require quite a bit of boilerplate code.
-
Imperative Style: You create a parser object and explicitly add arguments to it.
-
Composability: Can become complex when nesting commands (sub-parsers).
Deep Dive: click - The Elegant Contender
click is a popular third-party library designed to make creating beautiful and composable command-line interfaces easy, often with less code than argparse. It heavily utilizes decorators.
Installation:
pip install click
Core Concept: You decorate a Python function to turn it into a command. Decorators like @click.command()
, @click.argument()
, and @click.option()
define the CLI interface, and click automatically maps the parsed values to the function’s parameters.
Example: The Same Greeting Tool with click
Let’s rebuild greet.py
using click.
# greet_click.py
import click
# 1. Decorate the main function to make it a command
@click.command()
# 2. Define the 'name' positional argument
@click.argument('name')
# 3. Define the '--greeting' / '-g' option
@click.option(
'-g', '--greeting',
default='Hello',
help='The greeting phrase to use (default: Hello).'
)
# 4. Define the '--uppercase' / '-u' flag
@click.option(
'-u', '--uppercase',
is_flag=True, # Makes it a flag
help='Print the greeting in uppercase.'
)
def cli(name, greeting, uppercase):
"""A simple program that greets the user.""" # Docstring becomes help text!
message = f"{greeting}, {name}!"
if uppercase:
message = message.upper()
click.echo(message) # Use click.echo for better testability/compatibility
if __name__ == "__main__":
cli()
How to Run It:
Save this as greet_click.py
. The command-line usage is identical to the argparse
version!
# Basic usage
$ python greet_click.py Alice
Hello, Alice!
# Using options
$ python greet_click.py Bob --greeting "Good morning"
Good morning, Bob!
# Using flags
$ python greet_click.py Charlie -u
HELLO, CHARLIE!
# Combining
$ python greet_click.py David -g Welcome --uppercase
WELCOME, DAVID!
# Getting help (also automatically generated!)
$ python greet_click.py --help
Usage: greet_click.py [OPTIONS] NAME
A simple program that greets the user.
Arguments:
NAME [required]
Options:
-g, --greeting TEXT The greeting phrase to use (default: Hello).
-u, --uppercase Print the greeting in uppercase.
--help Show this message and exit.
Notice how the code with click is arguably cleaner and more declarative. The function signature def cli(name, greeting, uppercase): directly reflects the arguments and options.
click Pros:
-
Elegant Syntax: Decorator-based approach is often more readable and less verbose.
-
Composability: Excellent support for nesting commands using groups (@click.group()).
-
Sensible Defaults: Often does “the right thing” out of the box.
-
Extra Features: Built-in support for prompts, password inputs, progress bars, launching editors, etc.
-
Testability: Designed with testing in mind (e.g., CliRunner).
click Cons:
-
External Dependency: Requires pip install click.
-
Magic: Decorators can sometimes feel like magic if you don’t understand how they work.
-
Learning Curve: While simple cases are easy, mastering advanced features like context passing requires learning click’s specific concepts.
argparse vs. click: When to Choose Which?
Feature | argparse | click | Recommendation |
---|---|---|---|
Dependency | Built-in (Standard Library) | External (pip install click) | argparse if you cannot have external deps. click otherwise. |
Syntax | Imperative (create parser, add args) | Declarative (decorators) | click often feels cleaner, especially for complex interfaces. |
Boilerplate | More verbose | Less verbose | click requires less code for similar functionality. |
Composability | Sub-parsers can be complex | Excellent (@click.group) | click is generally better for tools with multiple sub-commands (like git). |
Features | Core parsing, types, help | Core parsing, types, help, prompts, colors, progress bars, etc. | click offers more batteries included. |
Ease of Use | Good for simple scripts | Easy for simple, scales well to complex | click often has a gentler learning curve for common patterns. |
General Guidance:
-
Use argparse if:
-
You need a CLI for a simple script.
-
You are strictly prohibited from adding external dependencies.
-
You prefer the explicit, imperative style.
-
-
Use click if:
-
You are building a more complex CLI, especially one with sub-commands.
-
You prefer the clean, declarative decorator syntax.
-
You want access to its additional features (prompts, colors, etc.) without writing them yourself.
-
Adding an external dependency is not an issue.
-
For most new projects where dependencies aren’t a blocker, click often leads to more maintainable and readable CLI code.
Best Practices for CLI Design
Regardless of the library you choose, follow these tips:
-
Clear Help Messages: Write descriptive help text for the command itself and each argument/option. Both libraries make this easy.
-
Sensible Defaults: Provide reasonable default values for options where possible.
-
Standard Conventions: Use standard flag names like -h/—help, -v/—version, -o/—output, -V/—verbose.
-
Positional vs. Optional: Use positional arguments for essential inputs the command cannot function without. Use options for everything else (modifying behavior, optional inputs).
-
Feedback: Let the user know what’s happening. Use print or click.echo. Consider a —verbose flag for more detailed output. Use progress bars for long tasks.
-
Error Handling: Catch potential errors (e.g., file not found) and provide informative error messages. Both libraries handle incorrect argument usage automatically.
-
Exit Codes: Use appropriate exit codes (0 for success, non-zero for errors) so your tool works well in scripts. sys.exit(1) for errors. click handles this automatically for parsing errors.
-
Keep it Focused: Follow the Unix philosophy – make your tool do one thing well. Combine tools using pipes if needed.
Making Your Tool Executable
To run your script without typing python first, you can make it directly executable:
-
Add a Shebang: Add this line at the very top of your Python script:
#!/usr/bin/env python3
This tells the operating system to execute the script using the Python 3 interpreter found in the user’s environment.
-
Set Execute Permissions: In your terminal, navigate to the directory containing your script and run:
chmod +x your_script_name.py
Now, you can run your script like this (assuming it’s in your current directory or PATH):
./greet_click.py Alice -u
# or if it's in your PATH
# greet_click.py Alice -u
Packaging and Distribution (Briefly)
For sharing your tool more widely, you’ll want to package it so others can install it using pip. This typically involves creating a pyproject.toml file (using tools like Poetry, Flit, or modern Setuptools) and defining an “entry point”.
An entry point links a command name (what the user types) to a function in your code (like the cli function in our click example).
Example snippet in pyproject.toml
(using Setuptools):
[project.scripts]
greet = "greet_click:cli"
After installing your package (pip install .), users could simply type greet Alice -u in their terminal from anywhere. Packaging is a bigger topic, but knowing about entry points is key for distributing CLI tools.
Conclusion
Building user-friendly CLI tools is a vital skill for any Python developer involved in automation, scripting, or creating developer utilities. While basic scripts might suffice initially, leveraging libraries like argparse or click elevates them into robust, reusable, and self-documenting applications.
argparse provides a solid, dependency-free foundation, while click offers a more modern, elegant, and feature-rich experience, especially for complex CLIs. Choose the one that best fits your project’s needs and constraints. Now go forth and turn those handy scripts into powerful command-line tools!
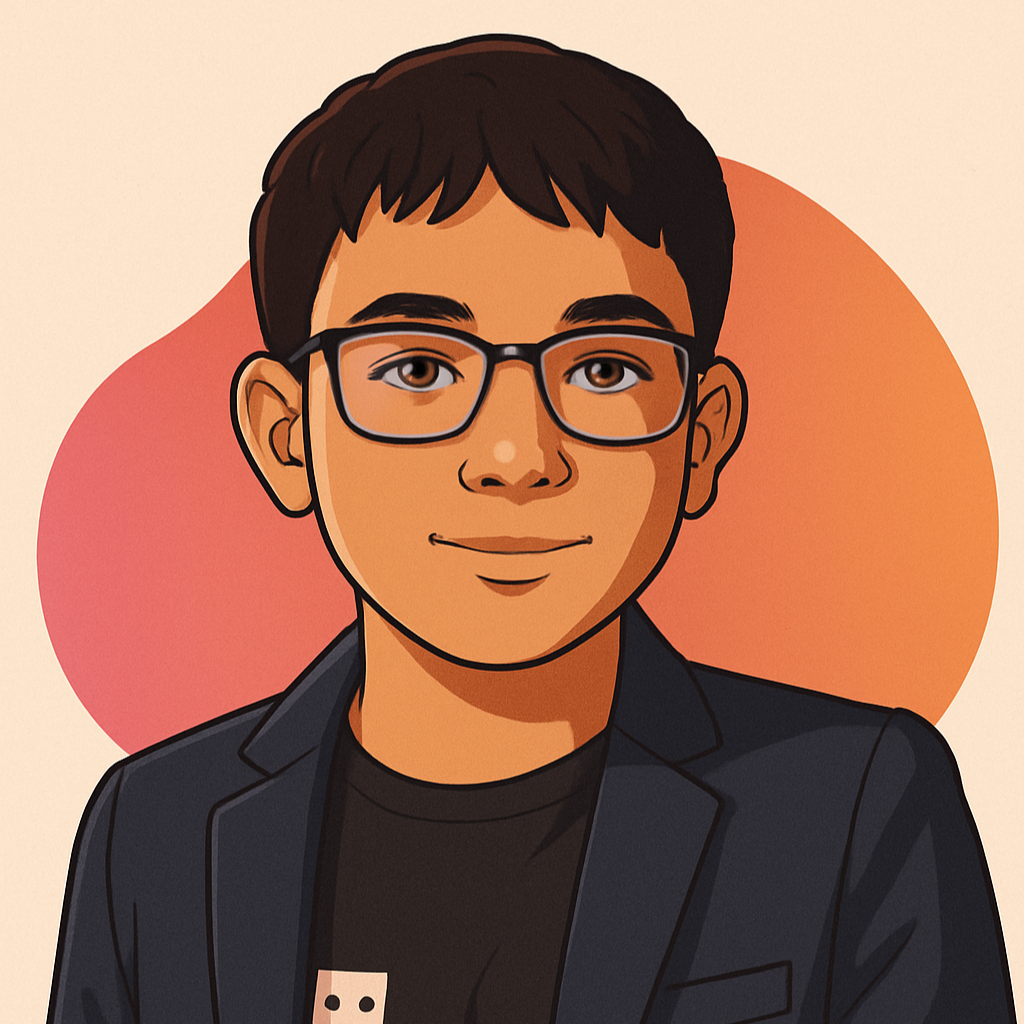
Solo Developer | Python & JS | FastAPI, Django, Next.js, Svelte