Mastering API Documentation with FastAPI: Leveraging Swagger UI & ReDoc
FastAPI has rapidly become a favorite Python web framework for building APIs, lauded for its speed, ease of use, and, crucially, its built-in support for automatic API documentation. Based on Python 3.7+ type hints, it generates OpenAPI and JSON Schema specifications for your API, providing interactive documentation interfaces right out of the box.
Manually documenting APIs is often tedious, error-prone, and struggles to keep pace with code changes. This guide dives deep into FastAPI’s automatic documentation features, powered by Swagger UI and ReDoc, showing you how to leverage them effectively to streamline your development workflow and create clear, usable APIs.
Table of Contents
- Why API Documentation Matters
- Installation and Setup
- FastAPI’s Auto-Documentation Magic
- Exploring the Interactive Docs
- How FastAPI Generates Documentation: The Pillars
- Benefits of Auto-Generated Documentation
- Customizing the Documentation
- Best Practices for Effective Documentation
- Conclusion
Why API Documentation Matters
Before diving in, let’s briefly touch upon why good API documentation is critical:
- Usability: Enables consumers (frontend developers, other backend services, third parties) to understand how to interact with your API effectively.
- Collaboration: Serves as a contract and communication tool between teams.
- Onboarding: Speeds up the process for new developers joining a project.
- Maintenance: Helps developers understand existing endpoints when making changes or debugging.
- Testing: Provides clear specifications for writing integration and end-to-end tests.
Manually maintaining this can be a significant drain on resources. FastAPI aims to solve this.
Installation and Setup
Core Dependencies
Ensure you have Python 3.7 or higher. You’ll need FastAPI and an ASGI server like Uvicorn.
pip install fastapi "uvicorn[standard]" pydantic
fastapi
: The core framework.uvicorn[standard]
: The ASGI server.[standard]
includes optional dependencies likeuvloop
andhttptools
for better performance.pydantic
: FastAPI uses Pydantic extensively for data validation and settings management, which is key to documentation generation. It’s usually installed as a dependency of FastAPI, but explicitly listing it is good practice.
Virtual Environments (Recommended)
It’s highly recommended to use a virtual environment to manage project dependencies:
# Create a virtual environment (e.g., named .venv)
python -m venv .venv
# Activate it (Linux/macOS)
source .venv/bin/activate
# Or (Windows PowerShell)
# .\.venv\Scripts\Activate.ps1
# Or (Windows Cmd)
# .venv\Scripts\activate.bat
# Now install packages inside the environment
pip install fastapi "uvicorn[standard]" pydantic
FastAPI’s Auto-Documentation Magic
FastAPI inspects your code, particularly type hints and function definitions, to generate an OpenAPI schema. OpenAPI (formerly Swagger Specification) is a standard, language-agnostic format for describing RESTful APIs. This schema then powers the visual documentation tools.
A Basic Example
Let’s create a simple FastAPI application in a file named main.py
:
# main.py
from fastapi import FastAPI
from pydantic import BaseModel
from typing import Optional
# Initialize the FastAPI app
# You can add metadata here that appears in the docs
app = FastAPI(
title="My Super API",
description="This is a very fancy API built with FastAPI",
version="1.0.0",
)
# --- Define Data Models (using Pydantic) ---
class Item(BaseModel):
name: str
description: Optional[str] = None
price: float
tax: Optional[float] = None
class CreateItemResponse(BaseModel):
item_id: int
item_data: Item
# --- Define API Endpoints (Path Operations) ---
@app.get("/")
def read_root():
"""
Root endpoint returning a simple welcome message.
Can be used as a health check.
"""
return {"message": "Welcome to My Super API!"}
@app.get("/items/{item_id}", tags=["Items"])
def read_item(item_id: int, query_param: Optional[str] = None):
"""
Retrieve details for a specific item by its ID.
- **item_id**: The unique identifier of the item (path parameter).
- **query_param**: An optional query parameter for filtering or modification.
"""
# In a real app, you'd fetch data from a database based on item_id
return {"item_id": item_id, "name": f"Sample Item {item_id}", "query_param": query_param}
@app.post("/items/", response_model=CreateItemResponse, status_code=201, tags=["Items"])
def create_item(item: Item):
"""
Create a new item.
Takes item details in the request body and returns the created item's ID
along with the provided data.
"""
# In a real app, you'd save the item to a database and get an ID
new_item_id = 123 # Dummy ID
print(f"Received item: {item.dict()}")
return {"item_id": new_item_id, "item_data": item}
Running the Application
Save the code as main.py
and run it with Uvicorn from your terminal:
uvicorn main:app --reload
main
: The Python file (main.py
).app
: The FastAPI instance object created insidemain.py
(app = FastAPI()
).--reload
: Tells Uvicorn to automatically restart the server when code changes are detected (great for development).
Uvicorn will start the server, typically on http://127.0.0.1:8000
.
Adding More Detail: Descriptions and Tags
Notice in the example:
FastAPI(...)
metadata: Thetitle
,description
, andversion
passed when creating theFastAPI
instance appear at the top of the documentation pages.- Docstrings: The docstrings within each path operation function (
read_root
,read_item
,create_item
) are used as the primary description for that endpoint. Markdown can be used here for formatting. tags
parameter: Addingtags=["Items"]
to the@app.get
and@app.post
decorators groups these related endpoints under an “Items” section in the UI, making navigation easier for larger APIs.
Working with Data: Request Body and Pydantic Models
The create_item
endpoint demonstrates how FastAPI handles request bodies and response models:
- Request Body (
item: Item
): By declaring the function parameteritem
with the type hintItem
(our Pydantic model), FastAPI knows to expect a JSON request body conforming to theItem
schema. This is automatically documented. - Response Model (
response_model=CreateItemResponse
): This parameter explicitly tells FastAPI (and the documentation) the structure of the expected successful response (HTTP 201 in this case). It helps with data serialization and clearly documents the output. - Status Code (
status_code=201
): Explicitly setting the default success status code ensures it’s correctly documented (default is 200 OK).
Exploring the Interactive Docs
With the server running, open your browser:
/docs
)
Swagger UI (Navigate to http://127.0.0.1:8000/docs
.
You’ll see the Swagger UI interface:
- Metadata: The API title, version, and description are displayed at the top.
- Grouping: Endpoints are grouped by tags (“Items” and “default” for
read_root
). - Endpoints: Each endpoint is listed with its HTTP method, path, and summary (first line of the docstring).
- Expansion: Clicking an endpoint reveals details:
- Full description (from the docstring).
- Parameters section (detailing path, query parameters, types, required status).
- Request Body section (for POST/PUT, showing the expected schema, often with an example).
- Responses section (showing possible status codes and the schema of the response body, like
CreateItemResponse
).
- “Try it out”: The key interactive feature. Clicking this allows you to fill in parameters/request bodies and execute the API call directly from the browser, seeing the actual request, response code, and response body.
/redoc
)
ReDoc (Navigate to http://127.0.0.1:8000/redoc
.

ReDoc offers an alternative view:
- Readability Focus: Often considered cleaner and more user-friendly for simply reading the documentation.
- Three-Panel Layout: Typically shows navigation on the left, detailed content in the center, and code examples/schemas on the right.
- Less Interactive: Does not include the “Try it out” functionality of Swagger UI.
- Customization: Supports features like dark mode.
Both interfaces are generated from the same underlying OpenAPI schema automatically produced by FastAPI.
How FastAPI Generates Documentation: The Pillars
FastAPI cleverly combines several Python features and its own components:
- Python Type Hints: The foundation. FastAPI inspects type hints for function parameters (
item_id: int
,query_param: Optional[str]
) and Pydantic model fields (name: str
,price: float
) to understand data types. - Pydantic Models: Used for data validation, serialization, and automatic JSON Schema generation. FastAPI converts Pydantic models into the JSON Schema definitions required by OpenAPI, documenting the structure of request bodies and response models.
- Path, Query, and Body Parameters: FastAPI analyzes function parameters:
- Parameters matching path variables (e.g.,
{item_id}
) become path parameters. - Parameters not in the path and having simple types (int, str, float, bool) become query parameters by default.
- Parameters whose type hint is a Pydantic model become request body parameters.
- Parameters matching path variables (e.g.,
- Docstrings: As seen, these are directly used for the descriptions of endpoints and can include Markdown.
- Status Codes: FastAPI infers defaults (200 for most, 201 for POST) or uses the
status_code
parameter in the decorator. Documenting various possible response codes (e.g., 404 Not Found) can be done using theresponses
parameter. - Tags: The
tags
list in decorators groups related endpoints. - Response Models: The
response_model
parameter defines the schema for the successful response body. - Dependencies: FastAPI’s dependency injection system (using
Depends
) can also integrate with documentation, indicating, for example, if an endpoint requires authentication declared via a dependency.
Benefits of Auto-Generated Documentation
- Synchronization: Documentation stays perfectly in sync with the code because it’s generated from the code.
- Reduced Effort: Dramatically cuts down manual documentation time and maintenance.
- Accuracy: Minimizes errors and discrepancies often found in manually written docs.
- Standardization: Adheres to the widely adopted OpenAPI standard.
- Interactivity: Swagger UI allows immediate testing and exploration.
- Collaboration: Provides a clear, shared understanding of the API contract for frontend, backend, and QA teams.
Customizing the Documentation
While the defaults are great, you can customize the docs:
- Change URLs: Modify the paths for Swagger and ReDoc:
app = FastAPI(docs_url="/api/docs", redoc_url="/api/redoc")
- Disable Docs: Set the URLs to
None
to disable them:app = FastAPI(docs_url=None, redoc_url=None)
- OpenAPI Metadata: Add more detail to the main
FastAPI
constructor (e.g.,contact
,license_info
). - Advanced OpenAPI: You can directly manipulate the generated OpenAPI schema for highly custom scenarios (refer to FastAPI advanced docs).
Best Practices for Effective Documentation
- Use Pydantic Models: Define clear request and response models using Pydantic for complex data structures.
- Be Liberal with Type Hints: Use precise type hints (
int
,str
,bool
,Optional
,List
, Pydantic models) everywhere. - Write Descriptive Docstrings: Explain what the endpoint does, its parameters, and any important side effects. Use Markdown for clarity. Add a concise summary on the first line.
- Utilize
tags
: Group related endpoints logically using tags. - Define
response_model
: Explicitly declare the success response structure. - Document Error Responses: Use the
responses
parameter in decorators to document potential error codes (e.g., 404, 422) and their schemas. - Add Examples: Use
Field
from Pydantic or theexamples
argument in path operation decorators to provide concrete examples in the documentation.
Conclusion
FastAPI’s automatic API documentation generation through Swagger UI and ReDoc is a game-changer. By leveraging Python’s type system and Pydantic, it transforms API documentation from a tedious chore into an integrated, accurate, and highly beneficial part of the development process. Embrace these features, follow best practices, and you’ll save significant time while producing APIs that are easier to understand, consume, and maintain. Happy coding!
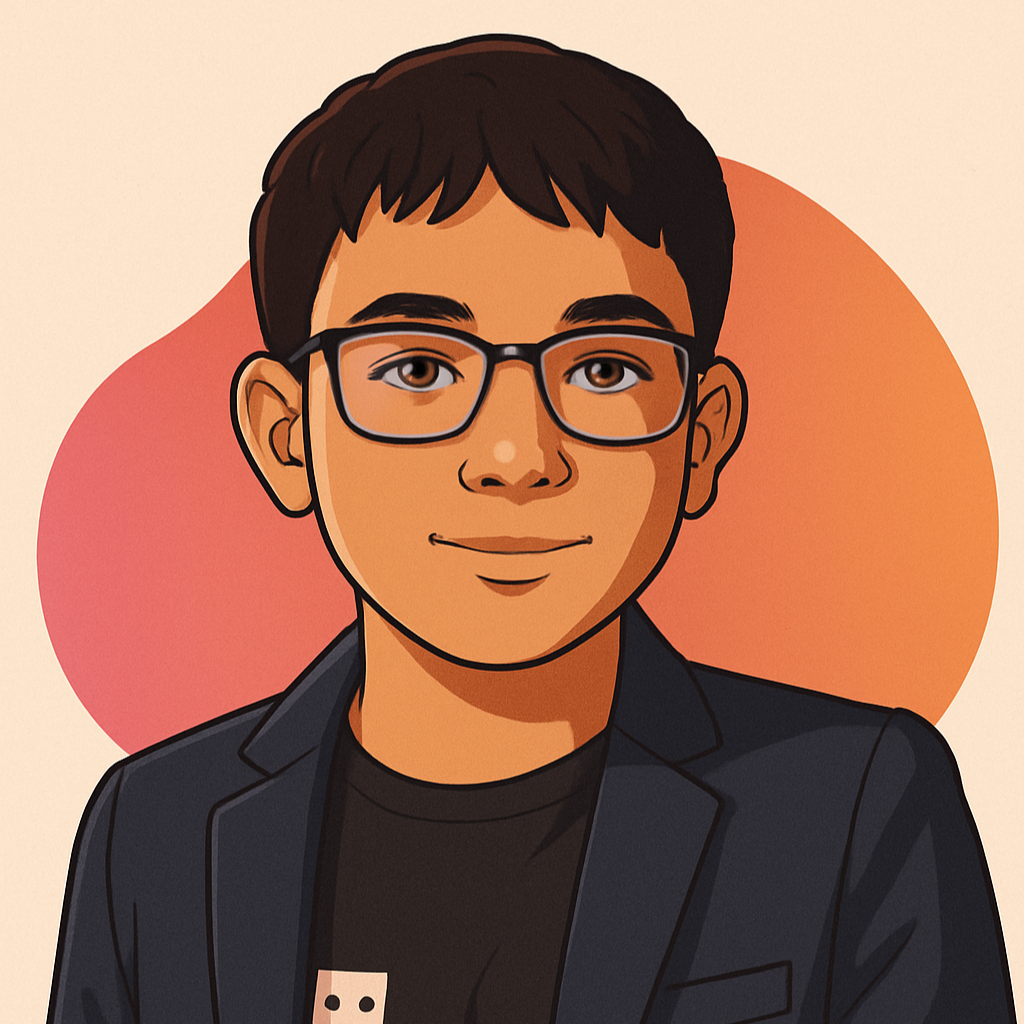
Solo Developer | Python & JS | FastAPI, Django, Next.js, Svelte