Regex Explained: A Beginner-Friendly Guide & Cheat Sheet
Whether you’re a seasoned developer or just starting out, Regular Expressions (Regex) can be an incredibly powerful tool in your programming toolkit. They might look cryptic at first, but this guide aims to demystify them step-by-step, with plenty of examples and a handy cheat sheet. Let’s dive in!
Table of Contents
- What is Regex (and Why Use It)?
- Core Concepts: The Building Blocks
- Basic Matching
- Quantifiers: How Many Times?
- Grouping and Capturing
- Escaping Special Characters (
\
) - Practical Examples (Explained)
- Regex Flags (Modifiers)
- Helpful Online Tools
- Conclusion & Next Steps
What is Regex (and Why Use It)?
Regex, short for Regular Expressions, is a sequence of characters that defines a search pattern. Think of it like a highly advanced “Find” feature (Ctrl+F or Cmd+F) on steroids.
Why use it?
- Validation: Check if input (like an email address or password) matches a required format.
- Searching: Find specific patterns within large amounts of text.
- Extraction: Pull out specific pieces of information (like phone numbers or URLs) from text.
- Manipulation: Find and replace text matching a pattern.
It’s a “secret code” used across many programming languages and tools (Python, JavaScript, Java, grep, text editors, etc.) to work efficiently with text data.
Core Concepts: The Building Blocks
Regex patterns are made up of two types of characters:
Literal Characters
Most characters simply match themselves. For example, the regex cat
will find the exact sequence of characters “c”, “a”, “t” in a string like “The cat sat on the mat”.
Metacharacters (Special Characters)
These characters have special meanings in regex. They don’t match themselves; instead, they perform specific functions. The main metacharacters are:
. \ + * ? [ ] ^ $ ( ) { } |
We’ll explore what each of these does. If you need to match one of these special characters literally, you need to “escape” it with a backslash \
(more on this later).
Basic Matching
Let’s look at the fundamental tools for matching characters.
.
) - Any Character
The Dot (- Matches any single character except for newline characters (
\n
). - Example:
h.t
matches “hat”, “hot”, “h t”, “h@t”, but not “ht” (needs a character in the middle) or “heat” (matches only one character).- Text: “hat hot hit heat ht”
- Matches found by
h.t
:hat
,hot
,hit
[...]
)
Character Classes (- Matches any single character that is listed inside the square brackets.
- Example:
gr[ae]y
matches “gray” or “grey”. It only matches one character from the seta
ore
.- Text: “Is it gray or grey?”
- Matches found by
gr[ae]y
:gray
,grey
- Ranges: You can specify ranges using a hyphen
-
.[a-z]
: Matches any lowercase letter.[A-Z]
: Matches any uppercase letter.[0-9]
: Matches any digit.[a-zA-Z]
: Matches any upper or lowercase letter.[a-zA-Z0-9]
: Matches any letter or digit.
- Example:
[0-9][a-z]
matches a digit followed by a lowercase letter, like “3a”, “0x”, “9z”.- Text: “file1a version9z dataX5”
- Matches found by
[0-9][a-z]
:1a
,9z
[^...]
)
Negated Character Classes (- The caret
^
inside square brackets means NOT. It matches any single character except those listed. - Example:
[^aeiou]
matches any single character that is not a lowercase vowel.- Text: “Rhythm”
- Matches found by
[^aeiou]
:R
,h
,y
,t
,h
,m
(each matched individually)
- Example:
q[^u]
matches a “q” not followed by a “u”.- Text: “Iraq Qatar Qi”
- Matches found by
q[^u]
:q
(in Iraq),Qi
\d
, \w
, \s
)
Predefined Character Classes (These are handy shortcuts for common character classes:
-
\d
: Matches any digit. Equivalent to[0-9]
. -
\D
: Matches any non-digit. Equivalent to[^0-9]
. -
\w
: Matches any “word character” (alphanumeric plus underscore). Equivalent to[a-zA-Z0-9_]
. -
\W
: Matches any non-word character. Equivalent to[^a-zA-Z0-9_]
. -
\s
: Matches any whitespace character (space, tab\t
, newline\n
, etc.). -
\S
: Matches any non-whitespace character. -
Example:
\d\d\d
matches exactly three digits, like “123”. -
Example:
\w+
matches one or more word characters (useful for finding words).- Text: “Hello_World-123!”
- Matches found by
\w+
:Hello_World
,123
^
, $
, \b
)
Anchors (Anchors don’t match characters; they match positions in the string.
-
^
: Matches the beginning of the string (or line, in multiline mode). -
$
: Matches the end of the string (or line, in multiline mode). -
\b
: Matches a “word boundary” – the position between a word character (\w
) and a non-word character (\W
) or the start/end of the string. -
\B
: Matches a non-word boundary. -
Example:
^Start
matches “Start” only if it’s at the very beginning of the string.- Text: “Start here” - Matches
Start
- Text: “Don’t Start” - No match
- Text: “Start here” - Matches
-
Example:
end$
matches “end” only if it’s at the very end of the string.- Text: “The end” - Matches
end
- Text: “The end.” - No match (unless
.
is matched explicitly)
- Text: “The end” - Matches
-
Example:
\bcat\b
matches the whole word “cat”.- Text: “The cat scattered.”
- Matches found by
\bcat\b
:cat
(it doesn’t match “cat” within “scattered” because ‘s’ and ‘e’ are word characters, so there’s no boundary).
Quantifiers: How Many Times?
Quantifiers specify how many times the preceding character, group, or character class must occur.
*
- Zero or More
- Matches the previous element 0 or more times.
- Example:
colou*r
matches “color” (0 ‘u’s) and “colour” (1 ‘u’). It could also match “colouuur” (many ‘u’s).- Text: “color colour colouur”
- Matches found by
colou*r
:color
,colour
,colouur
+
- One or More
- Matches the previous element 1 or more times.
- Example:
go+d
matches “god” (1 ‘o’) and “good” (2 ‘o’s), but not “gd” (0 ‘o’s).- Text: “god good gd”
- Matches found by
go+d
:god
,good
?
- Zero or One (Optional)
- Matches the previous element 0 or 1 time. Makes it optional.
- Example:
favou?rite
matches “favorite” (0 ‘u’s) and “favourite” (1 ‘u’).- Text: “favorite favourite”
- Matches found by
favou?rite
:favorite
,favourite
{n}
- Exactly n Times
- Matches the previous element exactly
n
times. - Example:
\d{3}
matches exactly three digits.- Text: “123 45 6789”
- Matches found by
\d{3}
:123
,678
(from 6789)
{n,}
- n or More Times
- Matches the previous element
n
or more times. - Example:
\d{2,}
matches two or more digits.- Text: “1 12 123 1234”
- Matches found by
\d{2,}
:12
,123
,1234
{n,m}
- Between n and m Times
- Matches the previous element at least
n
times, but no more thanm
times. - Example:
\w{3,5}
matches word characters that are 3, 4, or 5 characters long.- Text: “Go Run Fast Longer”
- Matches found by
\w{3,5}
:Run
,Fast
,Longe
(from Longer)
Greedy vs. Lazy Matching
By default, quantifiers (*
, +
, {n,}
, {n,m}
) are greedy. They try to match as much text as possible. Add a ?
after the quantifier to make it lazy, matching as little text as possible.
- Example Text:
<div>Content 1</div><div>Content 2</div>
- Greedy:
<div>.*</div>
matches<div>Content 1</div><div>Content 2</div>
(The.*
matches everything until the last</div>
) - Lazy:
<div>.*?</div>
matches<div>Content 1</div>
(first match) and<div>Content 2</div>
(second match). The.*?
stops at the first</div>
it encounters.
Grouping and Capturing
()
- Grouping & Capturing
Parentheses - Grouping: Treat multiple characters as a single unit, applying quantifiers to the whole group.
- Example:
(ha)+
matches “ha”, “haha”, “hahaha”, etc. The+
applies to the group(ha)
.
- Example:
- Capturing: Store the part of the string matched by the group for later use (e.g., extraction or backreferences). Captured groups are numbered starting from 1.
- Example: In
(\d{3})-(\d{4})
, group 1 captures the first 3 digits, and group 2 captures the last 4 digits from text like “123-4567”.
- Example: In
(?:...)
Non-Capturing Groups - Sometimes you need to group characters (e.g., for alternation or quantifiers) but you don’t need to capture the matched text. Using
(?:...)
is slightly more efficient and doesn’t clutter your capture results. - Example:
(?:Mr|Ms|Dr)\.?\s\w+
groups the titlesMr
,Ms
,Dr
for alternation, but doesn’t capture the matched title.
|
- OR Operator
Alternation - Matches either the expression before the pipe
|
OR the expression after it. Often used within groups. - Example:
cat|dog
matches “cat” or “dog”. - Example:
^(Error|Warning):
matches lines starting with “Error:” or “Warning:”.
\
)
Escaping Special Characters (If you want to match a character that has a special meaning in regex (like .
, *
, +
, ?
, ^
, $
, (
, )
, [
, ]
, {
, }
, |
, \
), you need to put a backslash \
before it. This is called “escaping”.
- Example: To match a literal dot
.
, use\.
. - Example: To match a literal backslash
\
, use\\
. - Example: To match
1+1=2
, the regex would be1\+1=2
.
Practical Examples (Explained)
Let’s break down some common regex patterns.
Example 1: Email Validation
^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$
^
: Start of the string.[a-zA-Z0-9._%+-]+
: One or more (+) allowed characters (letters, numbers,._%+-
) for the username part.@
: Literal ”@” symbol.[a-zA-Z0-9.-]+
: One or more (+) allowed characters (letters, numbers,.-
) for the domain name part.\.
: Literal ”.” symbol (escaped).[a-zA-Z]{2,}
: At least two ({2,}) letters for the top-level domain (like .com, .org).$
: End of the string.
Note: This is a common but simplified pattern. Truly comprehensive email validation (RFC 5322) is extremely complex.
Example 2: Date Matching (MM/DD/YYYY)
^(0[1-9]|1[0-2])\/(0[1-9]|[12]\d|3[01])\/\d{4}$
^
: Start of the string.(0[1-9]|1[0-2])
: Month - Captures group 1:0[1-9]
: Matches 01 to 09 OR1[0-2]
: Matches 10, 11, 12.
\/
: Literal ”/” (escaped, though often not strictly necessary).(0[1-9]|[12]\d|3[01])
: Day - Captures group 2:0[1-9]
: Matches 01 to 09 OR[12]\d
: Matches 10-19 or 20-29 OR3[01]
: Matches 30 or 31.
\/
: Literal ”/”.\d{4}
: Year - Exactly four digits.$
: End of the string.
Note: This pattern doesn’t validate correct days for each month (e.g., it allows 02/31).
Example 3: URL Extraction
https?:\/\/\S+
http
: Matches “http”.s?
: Matches the letter “s” zero or one time (making it optional, for http or https).:\/\/
: Matches ”://”.//
needs escaping.\S+
: Matches one or more non-whitespace characters (the rest of the URL).
Note: This is simple; real URLs can be more complex.
Example 4: Phone Number Extraction (Specific Format)
\b\d{3}-\d{3}-\d{4}\b
\b
: Word boundary (prevents matching inside longer numbers).\d{3}
: Exactly three digits (area code).-
: Literal hyphen.\d{3}
: Exactly three digits (prefix).-
: Literal hyphen.\d{4}
: Exactly four digits (line number).\b
: Word boundary.
Example 5: Finding Hashtags
#\w+
#
: Literal ”#” symbol.\w+
: One or more word characters (letters, numbers, underscore) following the hash.
Regex Flags (Modifiers)
Flags change how the regex engine interprets the pattern. They are usually specified outside the main pattern (e.g., /pattern/flags
). Common flags:
i
: Case-insensitive:cat
would match “cat”, “Cat”, “CAT”.g
: Global: Find all matches in the string, not just the first one.m
: Multiline: Makes^
and$
match the start/end of lines within the string (split by\n
), not just the absolute start/end of the entire string.s
(Dotall): Makes the dot (.
) match any character, including newline (\n
).
Helpful Online Tools
The best way to learn is by doing! These tools let you test patterns interactively:
- RegExr (regexr.com): Great visualization and explanations.
- RegEx101 (regex101.com): Detailed breakdown, supports multiple regex “flavors” (PCRE, Python, JS, etc.).
- RegexPlanet (regexplanet.com): Supports flavors for many different programming languages.
Use these tools to paste text, write your pattern, and see instantly what matches and why.
Conclusion & Next Steps
Regex is a dense but incredibly useful skill for anyone working with text. It might seem daunting initially, but breaking it down into smaller parts – characters, classes, quantifiers, anchors, groups – makes it manageable.
Don’t be afraid to experiment!
- Start with simple patterns.
- Use online tools extensively to test and debug.
- Try to solve small text problems you encounter (e.g., find all lines starting with “Date:”, extract numbers from a log file).
- Refer back to this cheat sheet often.
With practice, you’ll find regex becoming an indispensable part of your technical arsenal. Happy matching! 🚀🔍🎉
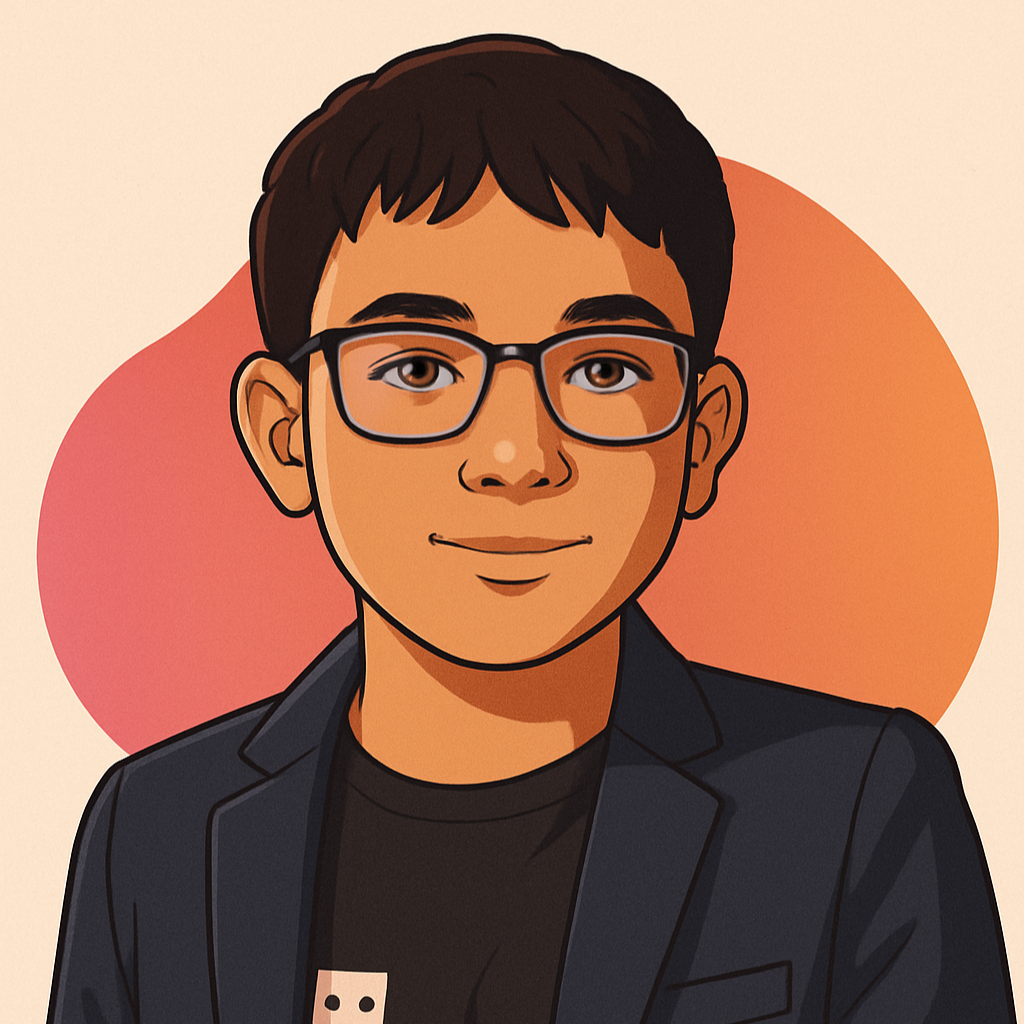
Solo Developer | Python & JS | FastAPI, Django, Next.js, Svelte