Demystifying Git and GitHub: A Comprehensive Beginner’s Guide to Version Control and Collaboration
In the fast-paced world of software development, effective collaboration and version control are pivotal to success. As coding projects evolve and multiple contributors work simultaneously, chaos can ensue without a robust system in place. This is where version control systems like Git and collaborative platforms like GitHub play a transformative role, bringing order, safety, and efficiency to the development process.
Table of Contents
- 1. Introduction
- 2. Getting Started with Git
- 3. Understanding Version Control Concepts
- 4. GitHub Essentials
- 5. Collaborative Workflows
- 6. Best Practices and Tips
- 7. Conclusion
1. Introduction
1.1 Why Version Control is Essential
Imagine working on a large document or project. How do you track changes? What if you make a mistake and need to go back to an older version? What if multiple people need to edit the file simultaneously without overwriting each other’s work? Version Control Systems (VCS) solve these problems for software code (and other digital files).
Key benefits include:
- History Tracking: See who changed what, when, and why.
- Reversibility: Easily revert files or the entire project back to a previous state.
- Branching & Merging: Work on different features or fixes independently and then combine them later.
- Collaboration: Enable multiple developers to work on the same project concurrently and efficiently merge their contributions.
- Backup: Distributed VCS like Git mean every collaborator has a full copy of the project history, providing inherent backup.
1.2 Overview of Git and GitHub
- Git: A free, open-source, distributed version control system created by Linus Torvalds (creator of Linux). It’s incredibly fast, efficient, and designed for managing projects of any size. “Distributed” means that you don’t rely on a central server; every developer’s computer holds a full copy (clone) of the repository, including its entire history. You work locally, committing changes, and then “sync” with remote repositories when needed.
- GitHub: A web-based platform built around Git. It provides hosting for Git repositories and adds features for collaboration, project management, and code sharing. Think of Git as the engine and GitHub as the car’s dashboard, body, and online community features. Key GitHub features include Pull Requests, issue tracking, code reviews, user management, and project boards. (Alternatives include GitLab, Bitbucket, etc.)
1.3 Importance of Version Control in Collaborative Coding
In team environments, Git and GitHub (or similar platforms) are indispensable. They provide a structured way to:
- Integrate code from multiple developers.
- Review code changes before they become part of the main codebase.
- Manage different versions of the software (e.g., development, staging, production).
- Maintain a clear history, making debugging and understanding project evolution much easier.
This guide will walk you through the fundamentals needed to start using these powerful tools effectively.
2. Getting Started with Git
Let’s get Git installed and configured on your machine.
2.1 Installing Git
(Installation instructions remain the same as the previous version - ensuring clarity for Windows, macOS, and Linux users).
- Windows: Download from git-scm.com, run installer, follow prompts (defaults are generally good).
- macOS: Check with
git --version
. Install/update via Homebrew (brew install git
) or download from git-scm.com. - Linux (Debian/Ubuntu):
sudo apt update && sudo apt install git
- Linux (Fedora):
sudo dnf install git
Verify installation: Open a new terminal/command prompt window after installation and type:
git --version
You should see the installed Git version number.
2.2 Configuring Git for the First Time
Git needs to know who you are to associate your identity with the commits you create. You only need to do this once per machine using the --global
flag.
Open a terminal or command prompt:
-
Set your username: This is the name that will appear in commit logs.
git config --global user.name "Your Full Name"
Example:
git config --global user.name "Alice Wonderland"
-
Set your email address: Use the email you’ll associate with your GitHub account.
git config --global user.email "your.email@example.com"
Example:
git config --global user.email "alice.wonderland@example.com"
-
(Optional) Verify configuration:
git config --list
Look for the
user.name
anduser.email
entries.
The --global
setting saves this configuration in a global Git configuration file for your user account, applying it to all Git projects you work on. You can also set configuration per-project by omitting --global
inside a specific repository directory.
2.3 Basic Git Commands and Workflow
Let’s walk through the most common workflow for a simple project:
Scenario: You’re starting a new project, creating a file, and saving its initial version.
-
Create a project directory:
mkdir my-git-project cd my-git-project
-
Initialize Git: Turn this directory into a Git repository.
git init
Output:
Initialized empty Git repository in /path/to/my-git-project/.git/
This creates the hidden.git
folder. -
Create a file: Use your text editor or the command line to create a file, e.g.,
README.md
.# Example using command line (Linux/macOS) echo "# My Awesome Project" > README.md # Or create and edit it with a text editor
-
Check the status: See what Git knows about your project.
git status
Output might look like:
On branch main No commits yet Untracked files: (use "git add <file>..." to include in what will be committed) README.md nothing added to commit but untracked files present (use "git add" to track)
Git sees
README.md
but isn’t tracking it yet (it’s “untracked”). -
Stage the file: Tell Git you want to include changes in
README.md
in your next commit. This moves it to the “Staging Area”.git add README.md
-
Check the status again:
git status
Output might look like:
On branch main No commits yet Changes to be committed: (use "git rm --cached <file>..." to unstage) new file: README.md
Now Git shows
README.md
as a “new file” ready to be committed. -
Commit the staged changes: Save the snapshot to the project history. The
-m
flag allows you to provide a commit message directly.git commit -m "Initial commit: Add README file"
Output includes commit details (branch, commit hash, message, files changed).
-
Check the status one last time:
git status
Output:
On branch main\nnothing to commit, working tree clean
This means your working directory matches the latest commit, and nothing is staged. -
View history: See the commit you just made.
git log
Output shows the commit hash, author, date, and message.
# For a more concise log git log --oneline
This status -> add -> commit
cycle is the fundamental Git workflow you’ll use constantly.
3. Understanding Version Control Concepts
Let’s delve deeper into the core ideas that make Git powerful.
3.1 Commits, Branches, and Merging
-
Commits (Snapshots): A commit is not just the changes; it’s a complete snapshot of all tracked files in your project at that moment, plus metadata (author, timestamp, message, pointer to the previous commit(s)). This sequence of linked commits forms the project’s history. Think of it like saving distinct versions of your entire project folder.
- Example Viewing Log:
# Default log git log # One line per commit git log --oneline # Fancy graph view (if available) git log --graph --oneline --decorate --all
- Example Viewing Log:
-
Branches (Pointers for Parallel Work): Imagine your project history as a timeline (the
main
branch). When you create a branch, you create a new pointer that starts at your current commit. As you make commits on this new branch, the pointer moves forward, creating a separate line of history. This lets you:-
Isolate Features: Develop a new feature without destabilizing the
main
branch. -
Fix Bugs Safely: Create a branch from a specific release version to fix a bug without including unrelated new features.
-
Experiment: Try out ideas without committing them to the main history unless they work.
-
Example Branching Scenario:
# You are on the 'main' branch git status # working tree clean # Start work on a new login feature git checkout -b feature/login # ... make changes to login files ... git add . git commit -m "Add basic login form structure" # ... make more changes ... git add . git commit -m "Implement password validation" # Urgent bug fix needed! Switch back to main (assuming it's clean) git checkout main # Create a branch for the bug fix git checkout -b hotfix/critical-bug # ... fix the bug ... git add . git commit -m "Fix critical bug #456" # Merge the hotfix back into main git checkout main git merge hotfix/critical-bug # Merge the fix git branch -d hotfix/critical-bug # Delete the hotfix branch # Now go back to working on the login feature git checkout feature/login # ... continue working ...
-
-
Merging (Combining Histories): The process of taking the independent line of development created on a feature branch and integrating its changes back into another branch (like
main
). Git compares the histories and tries to combine the work.-
Fast-Forward Merge: If the target branch (
main
) hasn’t changed since the feature branch was created, Git simply moves themain
pointer forward to the latest commit of the feature branch. Simple and clean. -
Three-Way Merge: If both branches have new commits, Git finds the common ancestor commit and creates a new merge commit that combines the changes from both branches. This merge commit has two parent commits.
-
Example Merge (after the scenario above, assuming login feature is done):
# Ensure login feature is complete and committed on feature/login branch # Switch to the target branch (main) git checkout main # Merge the feature branch into main git merge feature/login # Git might open an editor for you to confirm the merge commit message # Or, if conflicts occur, Git will pause here (see section 5.2) # Optionally delete the feature branch after successful merge git branch -d feature/login
-
3.2 Repository Structure
Visualizing the flow helps understand commands:
+-----------------------+ git add +-----------------+ git commit +-------------------+
| Working Directory | ---------------> | Staging Area | -------------------> | Repository |
| (Your project files) | <--------------- | (Index) | | (.git directory) |
| - Modified | git checkout | - Files staged | | - Commit History |
| - Untracked | (or reset) | for commit | | - Branches, Tags |
+-----------------------+ +-----------------+ +-------------------+
- Working Directory: Your active files. Changes here are not yet part of Git history.
- Staging Area: A “waiting room” or “drafting table”.
git add
selectively adds changes from the working directory here, preparing them for the next commit. This lets you craft commits precisely, including only related changes. - Repository: The permanent record stored in
.git
.git commit
takes everything in the staging area and creates a new permanent snapshot (commit) in the repository.
3.3 Forking and Cloning
Essential for using platforms like GitHub collaboratively:
-
Cloning:
git clone <url>
downloads an entire existing repository (usually from a remote server like GitHub) to your local machine. You get the files, all the history, and a connection back to the original URL (namedorigin
by default).- Example:
# Clone a project you want to contribute to or use git clone https://github.com/some-user/some-project.git cd some-project # Navigate into the cloned directory
- Example:
-
Forking (on GitHub/GitLab etc.): Clicking the “Fork” button on a repository’s webpage creates a new copy of that repository under your own account on the platform. It’s entirely separate from the original but retains a link back.
-
Why fork? If you don’t have direct write access to a repository, you fork it first. Then you
clone
your fork, make changes on a branch, push to your fork, and finally create a Pull Request from your fork back to the original repository to propose your changes. -
Example workflow with fork:
# 1. Go to github.com/original-owner/original-repo and click "Fork" # Now you have github.com/your-username/original-repo # 2. Clone YOUR fork to your local machine git clone https://github.com/your-username/original-repo.git cd original-repo # 3. Create a branch, make changes, commit, push to YOUR fork git checkout -b my-fix # ... edit files ... git add . git commit -m "Fix typo in README" git push origin my-fix # 4. Go back to GitHub and create a Pull Request from your fork's # 'my-fix' branch to the original repo's 'main' branch.
-
4. GitHub Essentials
Let’s focus on using GitHub to host repositories and collaborate.
4.1 Creating a GitHub Account
(Same as before - visit github.com, sign up.) Ensure you verify your email address. Consider enabling Two-Factor Authentication (2FA) for security.
4.2 Creating and Managing Repositories
- Creating on GitHub: Click “New repository”.
- Name: Choose a unique name (e.g.,
my-first-web-app
). - Description: Briefly explain the project.
- Public/Private: Public is visible to everyone; Private requires explicit access.
- Initialize with:
- README: Recommended. Creates a
README.md
file for project description. - .gitignore: Select a template (e.g., Python, Node) to auto-generate a file ignoring common temporary/generated files for that language.
- License: Choose an open-source license (e.g., MIT, Apache 2.0) if applicable.
- README: Recommended. Creates a
- Name: Choose a unique name (e.g.,
- Connecting Local to Remote (if local repo exists first):
# In your local project directory (already initialized with 'git init') # Add the GitHub repo URL as a 'remote' named 'origin' git remote add origin https://github.com/your-username/your-repo-name.git # Verify the remote was added git remote -v # Output should show 'origin' with fetch and push URLs # Rename the default local branch to 'main' (if needed, common practice now) # git branch -M main # Push the 'main' branch to 'origin', setting upstream tracking git push -u origin main # '-u' links your local 'main' to 'origin/main', so future 'git push'/'git pull' work simply
- Cloning an Existing GitHub Repo: If the repo already exists on GitHub and you want a local copy:
git clone https://github.com/your-username/your-repo-name.git cd your-repo-name
4.3 Pull Requests and Code Reviews
The standard GitHub collaboration model:
- Branch: Always create a new branch locally for your changes (
git checkout -b <branch-name>
). - Commit & Push: Make your commits on the branch and push the branch to the remote (
git push origin <branch-name>
). - Open Pull Request: Go to the repository on GitHub. You’ll likely see a banner suggesting creating a PR from your recently pushed branch. Click it, or go to the “Pull requests” tab and click “New pull request”.
- Select Branches: Ensure the base repository/branch (e.g.,
original-repo:main
) and head repository/branch (e.g.,your-fork:your-branch
) are correct. - Title & Description: Write a clear title and detailed description explaining the purpose and approach of your changes. Reference any relevant issues (e.g.,
Closes #123
). - Reviewers: Request reviews from specific team members or maintainers if applicable.
- Select Branches: Ensure the base repository/branch (e.g.,
- Code Review: Reviewers examine the “Files changed” tab in the PR. They can:
- Leave overall comments.
- Click on specific lines of code to add inline comments or suggestions.
- Approve the changes, request modifications, or reject the PR.
- Discussion & Updates: Engage in discussion via comments. If changes are needed, make more commits locally on the same branch and
git push
again. The PR updates automatically with the new commits. - Merge: Once approved and checks (like automated tests) pass, a maintainer merges the PR using the button on GitHub. Common merge options:
- Merge commit: Creates a merge commit, preserving branch history (default).
- Squash and merge: Combines all commits from the PR branch into a single commit on the base branch. Keeps history cleaner.
- Rebase and merge: Replays commits from the PR branch onto the base branch, creating a linear history (use with care).
- Clean Up: After merging, you can usually delete the feature branch on GitHub and locally (
git branch -d <branch-name>
). - Update Local: Switch back to your main branch and pull the merged changes:
git checkout main git pull origin main
5. Collaborative Workflows
Applying these concepts in a team setting.
5.1 Branching Strategies for Teams
(Explanation of Gitflow/GitHub Flow remains similar, emphasizing the why).
- Key Idea: Isolate work. Don’t commit directly to
main
. - GitHub Flow Example:
git checkout main
git pull origin main
(Get latest changes)git checkout -b feature/describe-the-feature
(Create new branch)- (Work: edit,
git add
,git commit
) git push origin feature/describe-the-feature
- Create PR on GitHub from
feature/describe-the-feature
tomain
. - Review, discuss, update, merge.
git checkout main
git pull origin main
(Get the merged feature)git branch -d feature/describe-the-feature
(Optional local cleanup)
5.2 Resolving Conflicts and Handling Merge Issues
When git merge
or git pull
fails due to conflicts:
- Git Tells You: The command output will list the conflicted files.
- Inspect Files: Open the conflicted files. Git inserts conflict markers:
<<<<<<< HEAD // Code from your current branch (e.g., main) console.log("Hello from main!"); ======= // Code from the branch being merged (e.g., feature/greeting) console.log("Greetings from the feature!"); >>>>>>> feature/greeting
- Edit to Fix: Manually edit the file to remove the
<<<<<<<
,=======
,>>>>>>>
markers and combine the code the way it should be. Decide which parts to keep, or rewrite the section entirely. Example Resolved Code:// Keeping both greetings, perhaps? console.log("Hello from main!"); console.log("Greetings from the feature!");
- Stage the Resolved File: Tell Git you’ve fixed the conflict in this file:
git add <the-conflicted-file.js>
- Repeat: Do this for all conflicted files.
- Commit the Merge: Once
git status
shows all conflicts are resolved and staged, commit:
Git usually pre-populates a merge commit message; you can often just save and close the editor. If it was agit commit
pull
that conflicted, the commit finishes the pull/merge operation.
5.3 Integrating Git into Daily Development Workflow
-
Stashing Changes: What if you need to switch branches quickly but have uncommitted changes you’re not ready to commit? Use
git stash
:# You have changes in your working directory, but need to switch branches git stash save "Work in progress on feature X" # Saves changes and cleans working dir # Now you can switch branches, pull changes, etc. git checkout main git pull # Ready to return to your work? git checkout feature/x-branch git stash pop # Applies the most recently stashed changes and removes the stash entry # Or `git stash apply` to apply without removing from stash list # `git stash list` shows saved stashes
-
Keeping History Clean (Before PR): Interactive rebase (
git rebase -i
) is powerful for tidying up your local commit history before sharing it in a PR. You can:squash
: Combine multiple small commits into one meaningful commit.reword
: Change commit messages.edit
: Amend a commit’s content.drop
: Delete a commit.reorder
: Change the order of commits.- Example: Combine the last 3 commits into one before pushing:
git rebase -i HEAD~3 # Your editor opens: change 'pick' to 'squash' (or 's') for the 2nd and 3rd commits. # Save and close. Another editor opens to write the new combined commit message. # Save and close again. History is rewritten locally. # Now push (might need --force-with-lease if you pushed before rebasing) git push --force-with-lease origin your-branch
Warning: Only rebase commits that haven’t been shared/pushed publicly or coordinate carefully with teammates if you must rebase a shared branch.
6. Best Practices and Tips
Following these guidelines leads to better collaboration and maintainability.
6.1 Writing Meaningful Commit Messages
(Explanation of convention remains similar - Subject, Blank Line, Body, Footer).
- Why? Helps others (and future you) understand why a change was made, speeding up debugging and reviews.
- Good Example:
feat: Add user password reset functionality Implement the complete password reset flow: - Request reset endpoint sending email with token. - Verification page checking token validity. - Endpoint to set new password using valid token. Closes #55
- Bad Example:
git commit -m "stuff"
orgit commit -m "Fix bug"
(Which bug? Why?)
6.2 Using .gitignore to Manage Unwanted Files
- Why?
- Keeps Repo Clean: Prevents committing generated files (build artifacts, compiled code), logs, editor backups, OS files (
.DS_Store
). - Avoids Conflicts: Prevents unnecessary merge conflicts on generated files.
- Reduces Repo Size: Avoids bloating the repository history with large binaries or dependencies.
- Security: Prevents accidentally committing files with sensitive credentials (
.env
, credentials files).
- Keeps Repo Clean: Prevents committing generated files (build artifacts, compiled code), logs, editor backups, OS files (
- How: Create a
.gitignore
file in the project root. Add patterns for files/directories to ignore. Each line is a pattern. Use#
for comments.- Example Patterns:
# Ignore specific file my_private_key.pem # Ignore all files with .log extension *.log # Ignore an entire directory node_modules/ # Ignore files in any directory named 'build' **/build/ # Don't ignore a specific file within an ignored directory (exception) !node_modules/important-module/keep_this.txt
.gitignore
itself to Git! (git add .gitignore
,git commit -m "Add gitignore"
). - Example Patterns:
6.3 Security Considerations in Version Control
(Explanation remains similar - Never commit secrets, use SSH/Tokens, review dependencies, manage permissions).
- Check Your Work: Before committing, use
git diff --staged
to review exactly what changes you are about to commit. Ensure no secrets slipped in. - Secrets Scanning: Platforms like GitHub offer automated secrets scanning to alert you if potential credentials are found in pushed code.
- Token Scopes: When creating GitHub Personal Access Tokens, grant only the necessary permissions (scopes) required for the task. Set expiration dates.
7. Conclusion
You’ve now journeyed through the essentials of Git and GitHub, from basic commands to collaborative workflows and best practices.
7.1 Recap of Key Concepts
(Recap points remain similar, reinforcing the core ideas covered).
- Version Control: Why it’s critical.
- Git: Local, distributed tracking (commits, branches, merges).
- GitHub: Remote hosting and collaboration platform (repos, PRs, issues).
- Workflow: The cycle of changing, staging, committing, pushing, pulling.
- Collaboration: Forking, branching, Pull Requests, code reviews, conflict resolution.
- Best Practices: Clear commits,
.gitignore
, security awareness.
7.2 Next Steps in Git Mastery
(Suggestions remain similar, encouraging further learning).
- Consistent Practice: The key to mastery is regular use.
- Advanced Git:
rebase
,cherry-pick
,stash
,reflog
,bisect
. - Team Workflows: Deep dive into Gitflow/GitHub Flow/GitLab Flow.
- Contribute: Find open-source projects that interest you.
- Automation: Explore GitHub Actions for CI/CD.
- Resources: Official Git/GitHub docs, Pro Git book (online free), interactive tutorials.
Remember, proficiency comes with experience. Don’t be afraid to experiment (especially on branches!), ask questions, and learn from mistakes. Git and GitHub are invaluable skills in modern development.
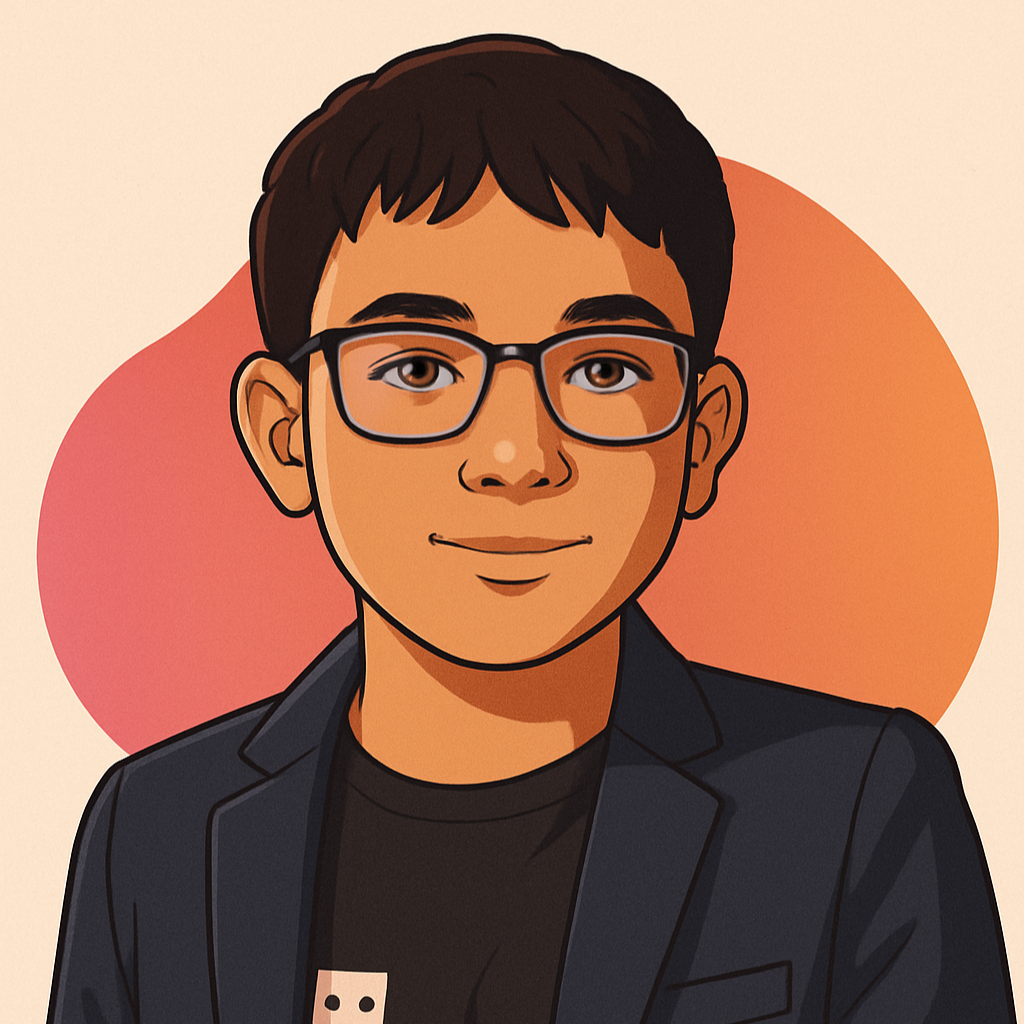
Solo Developer | Python & JS | FastAPI, Django, Next.js, Svelte