Mastering JavaScript Type Conversion: A Comprehensive Guide
JavaScript, the ubiquitous language of the web, is renowned for its flexibility and dynamic typing. A core concept underpinning this dynamism is type conversion (also known as type coercion). This is the process where JavaScript automatically or explicitly converts a value from one data type to another. For any web developer aiming to write clean, efficient, predictable, and bug-free code, a deep understanding of JavaScript’s type conversion mechanisms is not just helpful—it’s essential.
This guide will dive deep into the nuances of type conversion in JavaScript, exploring both implicit and explicit methods, common techniques, potential pitfalls, and best practices to help you wield this powerful feature effectively.
Table of Contents
- Understanding JavaScript Data Types
- Implicit vs. Explicit Type Conversion
- Common Type Conversion Techniques
- Key Pitfalls and Best Practices
- Conclusion: Gaining Control Through Understanding
Understanding JavaScript Data Types
Before tackling conversion, let’s refresh our understanding of JavaScript’s fundamental data types. They are broadly categorized into primitives and objects:
Primitive Data Types: (Immutable - their values cannot be changed)
number
: Represents both integer and floating-point numbers (e.g.,42
,3.14
). Includes special values likeInfinity
,-Infinity
, andNaN
(Not a Number).string
: Represents textual data, enclosed in single ('
), double ("
), or backticks (`
) (e.g.,"Hello World"
,'JavaScript'
,`Template literal`
).boolean
: Represents logical values:true
orfalse
.null
: Represents the intentional absence of any object value. It’s often assigned explicitly.undefined
: Represents a variable that has been declared but not yet assigned a value, or a function that doesn’t return anything.symbol
: (Introduced in ES6) Represents a unique and immutable identifier, often used as keys for object properties.bigint
: (Introduced in ES2020) Represents integers of arbitrary precision, useful for numbers beyond the safe integer limit ofnumber
.
Object Data Type: (Mutable - their values can be changed)
object
: Represents a collection of key-value pairs (properties and methods). This includes standard objects ({}
), arrays ([]
), functions (function(){}
),Date
,RegExp
, and more.
Understanding these types is the foundation for comprehending how values transition between them.
Implicit vs. Explicit Type Conversion
JavaScript performs type conversion in two ways:
1. Explicit Type Conversion (Type Casting): This occurs when the programmer intentionally converts a value’s type using built-in functions or operators. You are explicitly telling JavaScript, “I want this value as that type.”
- Achieved using: Functions like
String()
,Number()
,Boolean()
, methods like.toString()
,parseInt()
,parseFloat()
. - Example:
let str = "42"; let num = Number(str); // Explicitly convert the string "42" to the number 42 console.log(num); // Output: 42 console.log(typeof num); // Output: "number" let val = 0; let bool = Boolean(val); // Explicitly convert the number 0 to boolean false console.log(bool); // Output: false console.log(typeof bool); // Output: "boolean"
2. Implicit Type Conversion (Type Coercion): This happens automatically by JavaScript when operators are used with values of different types. JavaScript makes a “best guess” about what type is needed for the operation to proceed. This is powerful but can also lead to unexpected results if not understood well.
- Often occurs during: Arithmetic operations (
+
,-
,*
,/
,%
), comparisons (==
,!=
,>
,<
), logical operations (in some contexts), and string concatenation. - Example (Arithmetic):
let num = 5; // number let str = "2"; // string let result = num * str; // JS implicitly converts "2" to 2 before multiplying console.log(result); // Output: 10 console.log(typeof result); // Output: "number"
- Example (Comparison with
==
):console.log(5 == "5"); // Output: true (string "5" is implicitly converted to number 5 before comparison) console.log(true == 1); // Output: true (boolean true is converted to number 1) console.log(null == undefined); // Output: true (a specific rule of == coercion)
- Example (Addition
+
Behavior): The+
operator is special. If either operand is a string, it prefers string concatenation.let numA = 10; let numB = 5; let strC = "5"; console.log(numA + numB); // 15 (number + number = number) console.log(numA + strC); // "105" (number + string = string concatenation)
Implicit coercion is a key reason why understanding type conversion deeply is so important in JavaScript.
Common Type Conversion Techniques
Let’s explore the most frequent and useful ways to convert between types.
1. Converting to String
Needed when you want to represent a non-string value as text, often for display or concatenation.
- Using
String()
function: (Safest, works fornull
andundefined
)let numValue = 456; let boolValue = true; let nullValue = null; console.log(String(numValue)); // "456" console.log(String(boolValue)); // "true" console.log(String(nullValue)); // "null" console.log(String(undefined)); // "undefined"
- Using
.toString()
method: (Works for most types, but fails onnull
andundefined
)let numValue = 456; let boolValue = false; let arr = [1, 2]; console.log(numValue.toString()); // "456" console.log(boolValue.toString()); // "false" console.log(arr.toString()); // "1,2" // null.toString(); // Throws TypeError // undefined.toString(); // Throws TypeError
- Using String Concatenation (Implicit): Adding an empty string
""
coerces the other operand to a string.let numValue = 789; let result = "" + numValue; console.log(result); // "789" console.log(typeof result); // "string"
2. Converting to Number
Essential when dealing with input from forms (which are often strings), calculations, or data from APIs.
-
Using
Number()
function: (Stricter - converts the entire value, returnsNaN
if invalid)let numericString = "123.45"; let emptyString = ""; let spaceString = " 42 "; // Handles whitespace let invalidString = "12a"; let boolTrue = true; let nullVal = null; console.log(Number(numericString)); // 123.45 console.log(Number(emptyString)); // 0 console.log(Number(spaceString)); // 42 console.log(Number(invalidString)); // NaN (Not a Number) console.log(Number(boolTrue)); // 1 (true becomes 1, false becomes 0) console.log(Number(nullVal)); // 0 console.log(Number(undefined)); // NaN
-
Using
parseInt()
: (Parses from the beginning of the string until it hits a non-numeric character. Allows specifying radix/base.)let strWithPx = "123px"; let binaryStr = "1011"; let floatStr = "45.67"; console.log(parseInt(strWithPx)); // 123 (stops at 'p') console.log(parseInt(floatStr)); // 45 (stops at '.', integer part only) console.log(parseInt(binaryStr, 2)); // 11 (parses "1011" as base-2) console.log(parseInt("hello")); // NaN console.log(parseInt(" 50 ")); // 50 (handles whitespace)
Important: Always provide the
radix
(the base, usually 10 for decimal) toparseInt
to avoid unexpected behavior with strings starting with “0” or “0x”. E.g.,parseInt("010")
might be 8 or 10 depending on the environment if radix is omitted;parseInt("010", 10)
is reliably 10. -
Using
parseFloat()
: (Similar toparseInt
, but includes the decimal part.)let floatStr = "45.67abc"; let intStr = "89"; console.log(parseFloat(floatStr)); // 45.67 (stops at 'a') console.log(parseFloat(intStr)); // 89 console.log(parseFloat("3.14 meters")); // 3.14 console.log(parseFloat("hello")); // NaN
-
Using Unary Plus
+
operator (Implicit/Shorthand): A concise way to trigger number conversion, similar in behavior toNumber()
.let strNum = "99.9"; let boolVal = false; console.log(+strNum); // 99.9 (number) console.log(+boolVal); // 0 (number) console.log(+"hello"); // NaN
3. Converting to Boolean
Crucial for conditional logic (if
statements, ternary operators, loops). JavaScript has the concept of “truthy” and “falsy” values.
-
Falsy Values: These values coerce to
false
in a boolean context. There are only eight falsy values in JavaScript:false
0
(zero number)-0
(negative zero number)0n
(BigInt zero)""
(empty string)null
undefined
NaN
-
Truthy Values: Any other value not in the list above is considered “truthy” and coerces to
true
. This includes non-empty strings ("hello"
,"false"
), non-zero numbers (1
,-1
), objects ({}
), arrays ([]
), functions, etc. -
Using
Boolean()
function (Explicit):let truthyValue = "Hello"; let falsyValue = 0; let emptyObject = {}; // Truthy! let emptyArray = []; // Truthy! console.log(Boolean(truthyValue)); // true console.log(Boolean(falsyValue)); // false console.log(Boolean(emptyObject)); // true console.log(Boolean(emptyArray)); // true
-
Using Logical Contexts (Implicit):
if
statements, logical operators (&&
,||
,!
) trigger boolean conversion.let userName = ""; // Falsy if (userName) { // Implicitly converts userName to boolean (false) console.log("Username exists"); } else { console.log("Username is empty"); // This runs } let value = "some value"; // Truthy console.log(!value); // false (Logical NOT converts to boolean then negates)
-
Using the Double NOT Operator
!!
(Shorthand): See section 5.
4. Object to Primitive Conversion
This is a more complex conversion that happens when an object is used in a context expecting a primitive value (number or string). JavaScript tries a specific order to get a primitive representation:
[Symbol.toPrimitive](hint)
: If the object has this method (a newer standard), JavaScript calls it, passing a “hint” string:"number"
,"string"
, or"default"
. The method should return a primitive.valueOf()
: IfSymbol.toPrimitive
isn’t present or doesn’t return a primitive, and the hint is"number"
or"default"
, JavaScript tries callingvalueOf()
. If it returns a primitive, that value is used. By default, plain objects return themselves, arrays return themselves.toString()
: IfvalueOf()
doesn’t return a primitive (or if the hint was"string"
), JavaScript triestoString()
. If it returns a primitive, that value is used. Plain objects return"[object Object]"
, arrays return a comma-separated string of elements.
- Example (Default
toString
/valueOf
):let obj = { key: "value" }; let arr = [1, 2, 3]; console.log("Object: " + obj); // "Object: [object Object]" (toString called) console.log("Array: " + arr); // "Array: 1,2,3" (toString called) // console.log(10 + obj); // Throws TypeError in modern JS (usually), older might try valueOf then toString -> NaN
- Example (Custom
valueOf
):let customObj = { value: 42, valueOf: function() { console.log("valueOf called"); return this.value; }, toString: function() { console.log("toString called"); return `My value is ${this.value}`; } }; console.log(100 + customObj); // 142 (valueOf called because '+' prefers number) console.log("Info: " + customObj); // "Info: My value is 42" (toString called because '+' with string prefers string)
5. Using the Unary Plus (+) and Double NOT (!!) Operators
These are common shorthands leveraging implicit conversion rules:
-
Unary Plus
+
: As seen in the “Convert to Number” section, placing+
before a value is a concise way to explicitly trigger conversion to a number.let strVal = "50"; let numVal = +strVal; // Equivalent to Number(strVal) console.log(numVal); // 50 (number)
-
Double NOT
!!
: This is a quick way to explicitly convert any value to its boolean equivalent (true
orfalse
). The first!
coerces the value to a boolean and negates it; the second!
negates it back to the original boolean representation.let truthyValue = "Hello"; let falsyValue = ""; let numValue = 10; console.log(!!truthyValue); // true (Equivalent to Boolean(truthyValue)) console.log(!!falsyValue); // false (Equivalent to Boolean(falsyValue)) console.log(!!numValue); // true console.log(!!0); // false
Key Pitfalls and Best Practices
While powerful, JavaScript’s type conversion requires care:
-
==
vs===
: The abstract equality operator (==
) performs type coercion before comparison, leading to potentially confusing results (5 == "5"
is true). The strict equality operator (===
) checks both value and type without coercion (5 === "5"
is false). Best Practice: Almost always prefer===
for comparisons to avoid unexpected coercion bugs. Use==
only if you specifically need to allow coercion (e.g.,null == undefined
is a common, though debatable, use case). -
The
+
Operator Ambiguity: As shown,+
behaves differently depending on operand types (addition vs. concatenation). Best Practice: If performing arithmetic, ensure both operands are numbers before using+
. Explicitly convert usingNumber()
,parseInt()
,parseFloat()
, or unary+
if necessary.let inputA = "10"; let inputB = "5"; // Unsafe: // let sum = inputA + inputB; // "105" (string concatenation) // Safe: let sum = Number(inputA) + Number(inputB); // 15 (number addition) // Or: let sum = +inputA + +inputB; console.log(sum);
-
NaN
Propagation: Operations involvingNaN
usually result inNaN
.NaN
is also the only JavaScript value not equal to itself (NaN === NaN
isfalse
). Best Practice: Use theNumber.isNaN()
method to reliably check if a value isNaN
. Avoid the globalisNaN()
which has quirks (e.g.,isNaN("hello")
istrue
, which can be misleading). If a calculation might produceNaN
, check for it afterwards.let result = "abc" * 1; // result is NaN console.log(Number.isNaN(result)); // true (Correct way to check) // console.log(result === NaN); // false (Incorrect way to check)
-
parseInt()
Without Radix: Forgetting the radix (10
for decimal) can lead to errors if the string starts with0
(potentially interpreted as octal) or0x
(hexadecimal). Best Practice: Always specify the radix:parseInt(value, 10)
. -
Loss of Precision: Converting very large numbers or precise decimals between
number
andstring
can sometimes lead to precision loss due to the nature of floating-point representation. Be mindful when dealing with financial calculations or large identifiers (considerBigInt
or libraries for high precision). -
Over-Reliance on Implicit Coercion: While convenient, code filled with complex implicit coercions can become hard to read, understand, and debug. Best Practice: Favor explicit conversion (
Number()
,String()
,Boolean()
,!!
,+
) when the intent isn’t immediately obvious from the context. This makes your code more self-documenting and predictable. -
Truthy/Falsy Surprises: Remember that empty objects (
{}
) and empty arrays ([]
) are truthy. Don’t rely onif (myArray)
to check if an array has elements; useif (myArray.length > 0)
. -
Consider TypeScript: If managing types and avoiding coercion bugs becomes a significant challenge in a large project, consider using TypeScript. It adds static typing to JavaScript, catching many type-related errors during development rather than at runtime.
Conclusion: Gaining Control Through Understanding
JavaScript’s type conversion system is a double-edged sword. Its flexibility enables dynamic and concise code, but its implicit nature can introduce subtle bugs if not handled with awareness.
Mastering type conversion—knowing when and how JavaScript converts types implicitly, and how to perform explicit conversions safely—is a cornerstone of effective JavaScript development. By understanding the rules, leveraging explicit conversion for clarity, preferring strict equality (===
), and being mindful of the common pitfalls, you gain greater control over your code’s behavior. This leads to applications that are more robust, predictable, easier to debug, and ultimately more reliable.
Keep exploring these concepts, experiment in your browser’s console, and embrace the nuances of JavaScript’s type system. It’s a fundamental skill that will serve you well throughout your web development journey.
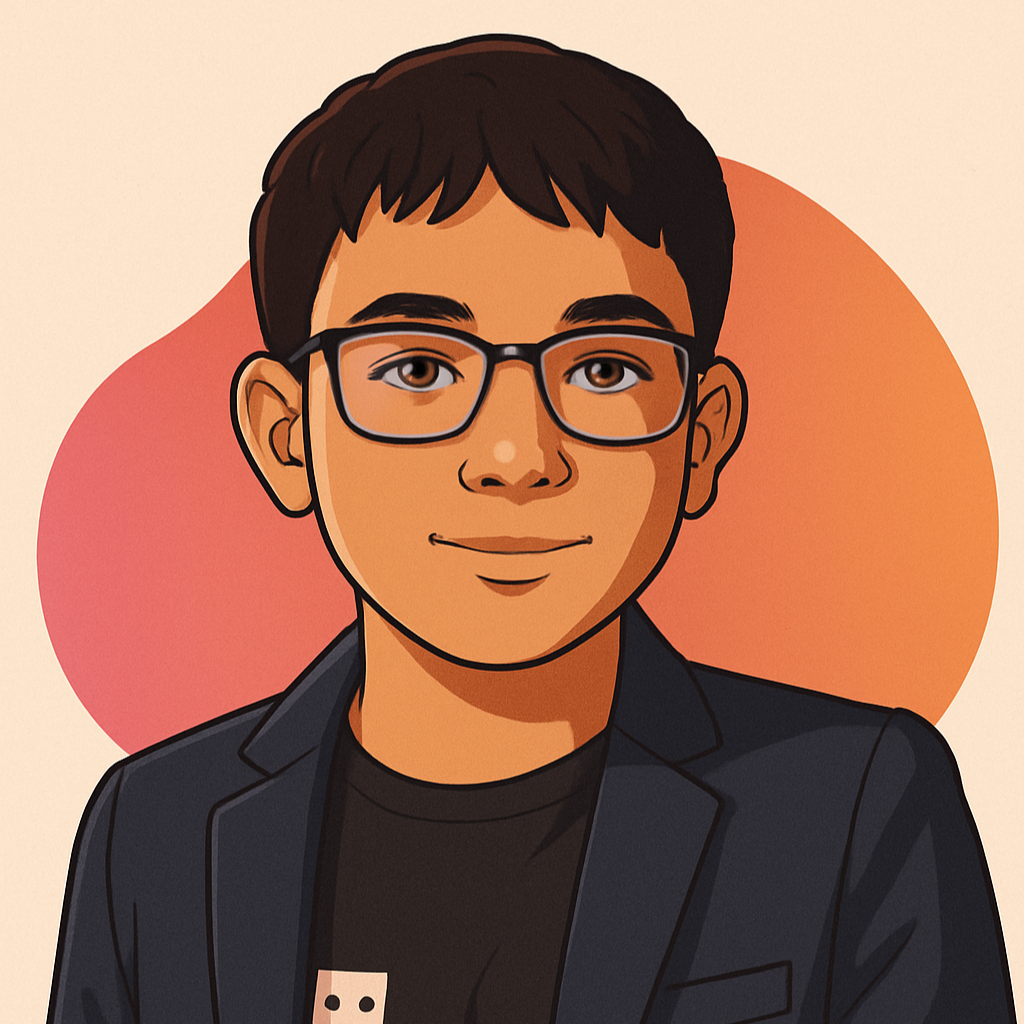
Solo Developer | Python & JS | FastAPI, Django, Next.js, Svelte