In the digital age, data is often called the new oil, and the vast expanse of the internet is its richest deposit. Web scraping, the automated process of extracting this data from websites, has become an indispensable skill for data scientists, researchers, market analysts, and developers. Python, renowned for its simplicity and powerful library ecosystem, stands out as a prime choice for web scraping tasks.
This post will guide you through the essentials of web scraping using Python, covering fundamental libraries like Requests
and BeautifulSoup
for static sites, and introducing Selenium
for handling dynamic, JavaScript-heavy pages. We’ll delve into setup, practical examples, and crucial best practices.
Table of Contents
- Understanding Web Scraping: What, Why, and the Rules of Engagement
- Setting Up Your Development Environment: Tools of the Trade
- Scraping Static Websites: Using Requests and BeautifulSoup
- Handling Dynamic Content: Introducing Selenium
- Essential Best Practices and Tips:** Scraping Responsibly and Effectively
- Conclusion: Your Next Steps in Web Scraping
Understanding Web Scraping
What is Web Scraping?
At its core, web scraping is the process of using bots (automated scripts) to fetch the content of web pages and then parse and extract specific pieces of information from the HTML structure. Instead of manually copying and pasting data, a scraper can perform this task rapidly and at scale.
Why Scrape the Web? Use Cases
Web scraping has numerous applications across various domains:
- Market Research: Tracking competitor pricing, product descriptions, and reviews.
- Data Analysis: Aggregating news articles, social media trends, or financial data.
- Lead Generation: Collecting contact information from directories or public profiles.
- Content Aggregation: Building news feeds or comparison websites.
- Academic Research: Gathering data from online journals, forums, or archives.
- Monitoring: Tracking website changes or online mentions.
Legal and Ethical Considerations
Before you start scraping, stop and consider the rules:
- Terms of Service (ToS): Most websites have a ToS page (often linked in the footer). Read it carefully. Many explicitly prohibit or restrict automated access or scraping. Violating the ToS can lead to IP blocks or legal action.
robots.txt
: This is a file located at the root of many websites (e.g.,https://example.com/robots.txt
). It provides instructions for web crawlers (like search engines and your scraper). Look forUser-agent:
lines (often*
for all bots) followed byDisallow:
directives. If a path you intend to scrape is listed underDisallow:
, you should not scrape it. While not legally binding, respectingrobots.txt
is a fundamental ethical practice.- Rate Limiting: Do not bombard a website with requests. This can overload their servers, impacting performance for other users and potentially causing a denial-of-service. Implement delays between your requests.
- Data Privacy: Be extremely cautious when dealing with personal data. Ensure compliance with privacy regulations like GDPR or CCPA. Avoid scraping sensitive or private information.
Scrape responsibly and ethically. If in doubt, err on the side of caution or seek permission.
Static vs. Dynamic Websites
- Static Websites: The HTML content is fully loaded with the initial page request. What you see when you “View Source” in your browser is generally what
requests
will receive. - Dynamic Websites: These sites use JavaScript to load or modify content after the initial HTML page is received. This could involve fetching data from APIs in the background or rendering content based on user interaction. Simple tools like
requests
won’t execute this JavaScript, meaning the data you want might be missing from the initial HTML source. This is where tools like Selenium become necessary.
Setting Up Your Development Environment
Installing Python
Ensure you have Python installed. You can download it from python.org. Python 3.7+ is recommended.
Virtual Environments
Using virtual environments is crucial for managing project dependencies and avoiding conflicts between different projects.
# Create a virtual environment named 'scrape_env' (or any name you prefer)
python -m venv scrape_env
# Activate the virtual environment
# On Linux/macOS:
source scrape_env/bin/activate
# On Windows (Command Prompt/PowerShell):
# scrape_env\Scripts\activate
You’ll see the environment name (e.g., (scrape_env)
) prefixed to your terminal prompt when it’s active.
Installing Core Libraries
With your virtual environment activated, install the necessary libraries using pip
:
pip install requests beautifulsoup4 selenium webdriver-manager
requests
: For making HTTP requests to fetch web pages.beautifulsoup4
(BS4): For parsing HTML and XML documents.selenium
: For automating web browser interaction, essential for dynamic sites.webdriver-manager
: A helpful utility to automatically download and manage the correct WebDriver executable for Selenium.
Requests
and BeautifulSoup
Scraping Static Websites: Using This approach works well for websites where the data you need is present in the initial HTML source code.
requests
)
Fetching Web Page Content (The requests
library makes sending HTTP requests straightforward.
import requests
url = "http://quotes.toscrape.com" # A site designed for scraping practice
try:
response = requests.get(url, timeout=10) # Add a timeout
response.raise_for_status() # Raise an exception for bad status codes (4xx or 5xx)
# Proceed if the request was successful
html_content = response.text # Get the HTML content as a string
# print(html_content[:500]) # Print the first 500 characters to check
except requests.exceptions.RequestException as e:
print(f"Error during requests to {url}: {e}")
# Handle error appropriately, maybe exit or retry
exit()
Understanding HTTP Status Codes
The response.status_code
tells you if your request was successful. Key codes include:
200 OK
: Success!3xx Redirection
: You might need to handle redirects.requests
often does this automatically.4xx Client Error
: You did something wrong (e.g.,404 Not Found
,403 Forbidden
- maybe blocked).5xx Server Error
: The website’s server had a problem.
Using response.raise_for_status()
is a convenient way to automatically check for 4xx
and 5xx
errors.
BeautifulSoup
)
Parsing HTML (Once you have the HTML content, BeautifulSoup
helps you navigate and search the structure.
from bs4 import BeautifulSoup
soup = BeautifulSoup(html_content, "html.parser")
# 'html.parser' is Python's built-in parser. 'lxml' is faster but needs separate installation.
Inspecting Browser Elements
Before you can extract data, you need to know where it is in the HTML. Use your browser’s Developer Tools (usually opened by pressing F12 or right-clicking on an element and selecting “Inspect” or “Inspect Element”):
- Identify the Target: Locate the data you want on the web page.
- Inspect: Right-click on it and choose “Inspect.”
- Analyze: The Developer Tools will highlight the corresponding HTML code. Look for unique tags,
id
attributes (usually unique),class
attributes (can be shared), or the structure surrounding the element. This tells you how to select it with BeautifulSoup.
Extracting Data
BeautifulSoup offers several ways to find elements:
- By Tag:
soup.find('h1')
(finds the first<h1>
tag),soup.find_all('p')
(finds all<p>
tags). - By Attributes:
soup.find(id='main-content')
,soup.find_all('span', class_='text')
. Note the underscore inclass_
becauseclass
is a reserved keyword in Python. - CSS Selectors: Often the most flexible method.
soup.select_one('.author')
(finds the first element with class ‘author’),soup.select('div.quote span.text')
(finds all<span>
tags with class ‘text’ inside<div>
tags with class ‘quote’).
Example: Scraping Quotes
Let’s refine the initial example using best practices and CSS Selectors.
import requests
from bs4 import BeautifulSoup
import csv # To save data
url = "http://quotes.toscrape.com"
headers = { # Pretend to be a browser
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Safari/537.36'
}
scraped_data = []
try:
response = requests.get(url, headers=headers, timeout=10)
response.raise_for_status() # Check for HTTP errors
soup = BeautifulSoup(response.text, "html.parser")
# Find all divs containing quote information
quote_elements = soup.select("div.quote") # Select all divs with class 'quote'
if not quote_elements:
print("No quotes found. Website structure might have changed.")
else:
for quote_element in quote_elements:
# Extract text using CSS selectors within each quote div
text = quote_element.select_one("span.text")
author = quote_element.select_one("small.author")
tags = quote_element.select("a.tag") # Find all tag links
# Get text content, handling potential missing elements
quote_text = text.get_text(strip=True) if text else "N/A"
author_name = author.get_text(strip=True) if author else "N/A"
tag_list = [tag.get_text(strip=True) for tag in tags]
print(f'"{quote_text}" - {author_name} (Tags: {", ".join(tag_list)})')
scraped_data.append([quote_text, author_name, ", ".join(tag_list)])
except requests.exceptions.RequestException as e:
print(f"Error during requests to {url}: {e}")
# Example: Save to CSV
if scraped_data:
try:
with open('quotes.csv', 'w', newline='', encoding='utf-8') as csvfile:
writer = csv.writer(csvfile)
writer.writerow(['Quote', 'Author', 'Tags']) # Write header
writer.writerows(scraped_data)
print("\nData successfully saved to quotes.csv")
except IOError as e:
print(f"Error writing to CSV file: {e}")
Handling Dynamic Content: Introducing Selenium
Why Selenium? The Role of JavaScript
When data is loaded via JavaScript after the initial page load, requests
won’t see it because it doesn’t execute JavaScript. Selenium
automates a real web browser (like Chrome or Firefox), which does execute JavaScript, allowing you to access the fully rendered page content.
Setting Up Selenium and WebDriver
Selenium requires a WebDriver executable that matches your browser and version. webdriver-manager
simplifies this:
from selenium import webdriver
from selenium.webdriver.chrome.service import Service
from webdriver_manager.chrome import ChromeDriverManager
# Automatically downloads/manages ChromeDriver and sets up the service
service = Service(ChromeDriverManager().install())
# Initialize the Chrome driver
driver = webdriver.Chrome(service=service)
# You can also use Firefox: webdriver.Firefox(service=Service(GeckoDriverManager().install()))
Automating Browser Actions
Selenium can do more than just fetch pages:
driver.get(url)
: Navigates the browser to a URL.driver.find_element(By.ID, 'element_id')
: Finds a single element.driver.find_elements(By.CLASS_NAME, 'element_class')
: Finds multiple elements.- (Other
By
options includeNAME
,TAG_NAME
,CSS_SELECTOR
,XPATH
,LINK_TEXT
) element.click()
: Clicks an element (e.g., a button).element.send_keys("some text")
: Types text into an input field.driver.page_source
: Gets the HTML source of the currently rendered page.
Waiting Strategies (Explicit Waits vs. time.sleep())
Avoid time.sleep()
where possible! Fixed waits are unreliable. If the content loads faster, you waste time. If it loads slower, your script might fail because the element isn’t ready yet.
Use Explicit Waits: Tell Selenium to wait for a specific condition to be met before proceeding (e.g., an element becoming visible or clickable). This is much more robust.
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
from selenium.common.exceptions import TimeoutException
try:
# Wait up to 10 seconds for elements with class 'quote' to be present
wait = WebDriverWait(driver, 10)
quote_elements_present = wait.until(EC.presence_of_all_elements_located((By.CSS_SELECTOR, "div.quote")))
print("Page loaded and quotes found!")
# Now you can safely extract data from driver.page_source or Selenium elements
except TimeoutException:
print("Loading took too much time or quotes not found!")
Extracting Dynamically Loaded Data
Once the dynamic content has loaded (verified using waits), you can get the rendered HTML using driver.page_source
and parse it with BeautifulSoup
as before, or you can directly work with the Selenium WebElement
objects found using driver.find_element(s)
.
Example: Scraping Dynamically Loaded Content (Conceptual)
Let’s adapt the quote scraper for a hypothetical dynamic version where quotes load after a delay.
from selenium import webdriver
from selenium.webdriver.chrome.service import Service
from webdriver_manager.chrome import ChromeDriverManager
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
from selenium.common.exceptions import TimeoutException, NoSuchElementException
from bs4 import BeautifulSoup
import time
# Hypothetical URL where quotes load dynamically
url = "http://quotes.toscrape.com/js/" # This page actually uses JS for loading
service = Service(ChromeDriverManager().install())
driver = webdriver.Chrome(service=service)
driver.maximize_window() # Often helpful
scraped_data = []
try:
driver.get(url)
print("Waiting for dynamic content to load...")
# Wait for the *container* of the quotes or a specific quote element to appear
wait = WebDriverWait(driver, 15) # Increase wait time if needed
wait.until(EC.presence_of_element_located((By.CSS_SELECTOR, "div.quote")))
print("Quotes container found.")
# Optional: Handle pagination if quotes load on scroll or button click
# while True: # Loop for pagination
# try:
# Find and click the 'Next' button
# next_button = wait.until(EC.element_to_be_clickable((By.CSS_SELECTOR, "li.next > a")))
# next_button.click()
# print("Clicked 'Next', waiting for new quotes...")
# time.sleep(2) # Give a brief moment for JS to potentially load
# Add a wait here for a *new* element that appears after clicking next
# wait.until(...)
# except TimeoutException:
# print("No more 'Next' button found or it timed out.")
# break # Exit pagination loop
# except NoSuchElementException:
# print("No 'Next' button found.")
# break
# Once content (or all pages) loaded, get the final page source
final_html = driver.page_source
soup = BeautifulSoup(final_html, "html.parser")
# Extract data using BeautifulSoup as before
quote_elements = soup.select("div.quote")
if not quote_elements:
print("No quotes found in the final source.")
else:
for quote_element in quote_elements:
text = quote_element.select_one("span.text")
author = quote_element.select_one("small.author")
tags = quote_element.select("a.tag")
quote_text = text.get_text(strip=True) if text else "N/A"
author_name = author.get_text(strip=True) if author else "N/A"
tag_list = [tag.get_text(strip=True) for tag in tags]
print(f'"{quote_text}" - {author_name}') # Print as we find
scraped_data.append([quote_text, author_name, ", ".join(tag_list)])
except TimeoutException:
print(f"Timed out waiting for page elements on {url}")
except Exception as e: # Catch other potential Selenium/general errors
print(f"An error occurred: {e}")
finally:
print("Closing browser.")
driver.quit() # ALWAYS close the driver to free up resources
# Process/save scraped_data here...
print(f"\nSuccessfully scraped {len(scraped_data)} quotes.")
Essential Best Practices and Tips
Respect robots.txt
Always check domain.com/robots.txt
before scraping. Honor the Disallow
directives for the user agents specified (or *
for all).
Identify Your Bot (User-Agents)
By default, requests
might send a generic User-Agent. Some websites block non-browser user agents. It’s good practice to set a realistic browser User-Agent or even a custom one identifying your bot (e.g., MyCoolScraperBot/1.0 (+http://mywebsite.com/botinfo)
).
headers = {
'User-Agent': 'Mozilla/5.0 (compatible; MyScraperBot/1.1; +http://example.com/bot)'
}
response = requests.get(url, headers=headers)
For Selenium, the browser sends its own native User-Agent.
Implement Delays and Rate Limiting
Don’t hit the server too rapidly. Use time.sleep(seconds)
between requests. A delay of 1-5 seconds is often reasonable, but adjust based on the website’s responsiveness and robots.txt
(which might specify a Crawl-delay
). Consider implementing exponential backoff if you get blocked (wait longer after each failure).
Robust Error Handling
Websites change, networks fail. Wrap your scraping logic in try...except
blocks. Catch specific exceptions like requests.exceptions.RequestException
, TimeoutException
, NoSuchElementException
(Selenium), AttributeError
(BeautifulSoup, if an element is missing), etc. Log errors properly.
Handle Website Structure Changes
Scrapers are brittle. If the website updates its HTML structure (e.g., changes class names), your scraper will likely break. Be prepared to maintain and update your selectors. Using more stable selectors (like IDs if available, or less nested CSS selectors) can help slightly.
Use Logging Effectively
Instead of print()
, use Python’s built-in logging
module. It allows you to:
- Log different levels of information (DEBUG, INFO, WARNING, ERROR, CRITICAL).
- Log timestamps and other contextual info.
- Easily write logs to files for later analysis.
- Control log output verbosity.
Consider Data Storage
Think about how you’ll store the extracted data. Options include:
- CSV: Simple, human-readable, good for tabular data.
- JSON: Good for nested or less structured data, easy to parse in many languages.
- Database (SQLite, PostgreSQL, MySQL, MongoDB): Best for large datasets, complex relationships, or ongoing scraping tasks. Libraries like
SQLAlchemy
(for SQL) orpymongo
(for MongoDB) help interact with databases. Pandas DataFrames are also excellent for intermediate processing and saving to various formats.
Check for APIs First
This is the most important tip. Before scraping, check if the website offers a public API (Application Programming Interface). APIs provide structured data directly, are more stable, faster, and represent the intended way for programmatic access. Look for links like “API,” “Developers,” or monitor network requests in your browser’s Developer Tools (XHR/Fetch tab) to see if the website itself uses internal APIs to load data dynamically – you might be able to use those directly (check terms!).
Headless Browsing with Selenium
When running Selenium scripts on servers or without needing to see the browser window, use “headless” mode. It runs the browser in the background, saving resources.
from selenium.webdriver.chrome.options import Options
chrome_options = Options()
chrome_options.add_argument("--headless")
chrome_options.add_argument("--window-size=1920,1080") # Often needed in headless
service = Service(ChromeDriverManager().install())
driver = webdriver.Chrome(service=service, options=chrome_options)
# ... rest of your Selenium code
Conclusion: Your Next Steps in Web Scraping
Web scraping with Python is a potent technique for unlocking the web’s data. We’ve covered the fundamentals using Requests
and BeautifulSoup
for static sites and Selenium
for dynamic ones, emphasizing ethical considerations and robust practices.
Remember:
- Be Ethical: Respect
robots.txt
and ToS. Don’t overload servers. - Be Robust: Implement waits, error handling, and logging.
- Be Adaptable: Websites change; maintain your scrapers.
- Prefer APIs: Always look for an official API first.
The journey doesn’t end here. Explore libraries like Scrapy
(a full-fledged scraping framework), advanced parsing with lxml
and XPath, handling CAPTCHAs (ethically!), and deploying scrapers to the cloud. Continue learning, practice responsibly, and harness the power of web data effectively.
Happy scraping!
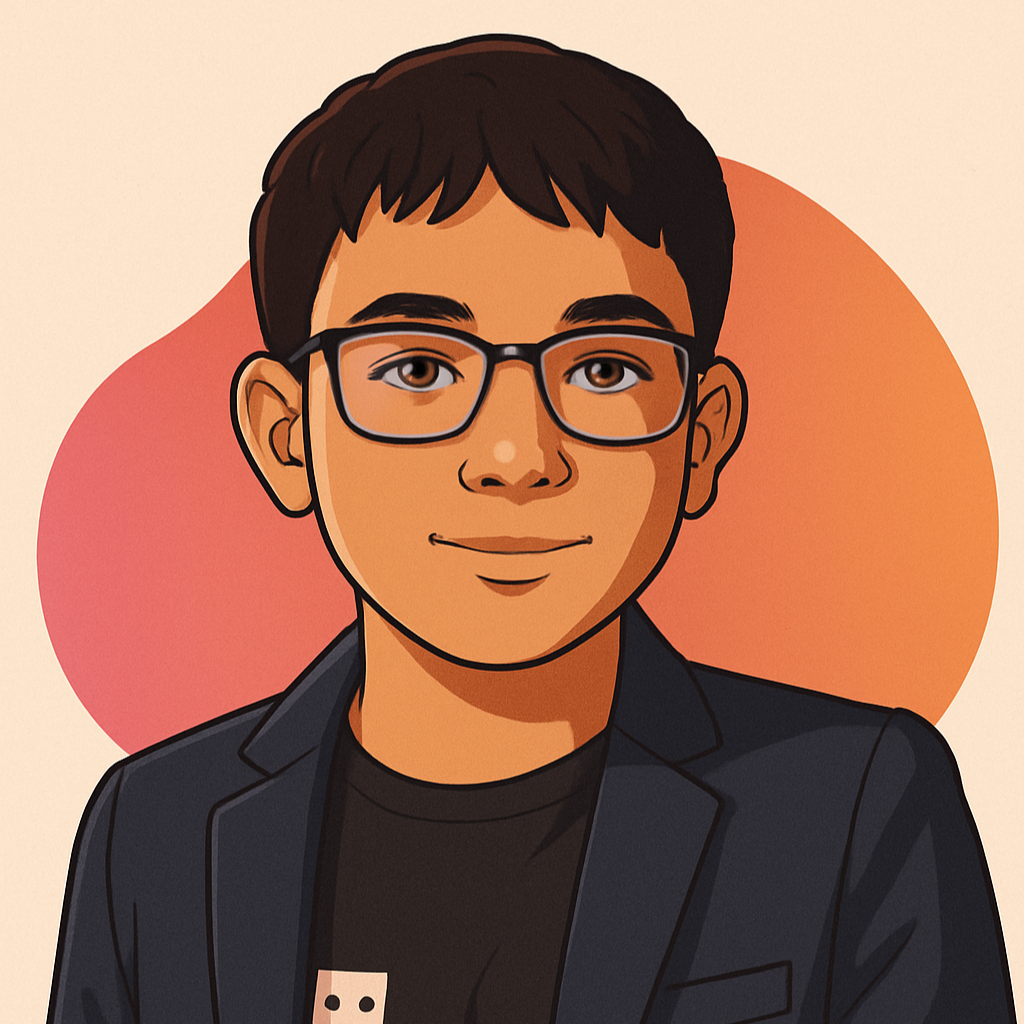
Solo Developer | Python & JS | FastAPI, Django, Next.js, Svelte