Python Threading Explained: Enhancing Concurrent Programming
In concurrent programming, threading plays a pivotal role in enhancing the efficiency of programs by allowing them to perform multiple tasks simultaneously. Python, a versatile and widely-used programming language, provides a threading
module that enables developers to leverage the power of concurrent execution.
Table of Contents
- Introduction
- Basics of Threading
- Thread Synchronization
- Thread Communication
- Thread Safety
- Advanced Threading Concepts
- Common Pitfalls in Threading and How to Avoid Them
- Case Studies: Real-world Examples of Python Threading
- Conclusion
Introduction
1.1 Overview of Threading in Python
Threading involves the execution of multiple threads (smaller units of a process) concurrently, enabling better resource utilization and improved responsiveness. Python‘s threading
module facilitates the creation, synchronization, and communication between threads, offering a robust foundation for building concurrent applications.
1.2 Importance of Threading in Concurrent Programming
Concurrent programming addresses the challenge of efficiently handling multiple tasks concurrently. Threading, as a concurrency model, allows developers to design programs that can perform several operations concurrently, enhancing the overall performance and responsiveness of applications. This section delves into why threading is crucial in the context of Python programming, laying the groundwork for the subsequent exploration of Python’s threading capabilities.
Basics of Threading
Threading in Python involves the execution of multiple threads within a single process, allowing for parallel execution of tasks. Understanding the basics of threading is fundamental for harnessing the full potential of concurrent programming in Python.
2.1 Understanding Threads and Processes
A thread is the smallest unit of execution within a process. Unlike processes, threads share the same memory space, making it more efficient for communication between them. This section provides an introduction to threads and highlights the differences between threads and processes.
Threads vs. Processes
- Threads: Lightweight, share the same memory space, and are suitable for tasks that can benefit from parallel execution.
- Processes: Independent memory space, heavier in terms of resource consumption, and are used for tasks that require a high degree of isolation.
2.2 Creating and Starting Threads
Creating and starting threads in Python involves using the threading
module. Here’s a basic example demonstrating how to create and start a thread:
import threading
import time
# Function to be executed in the thread
def print_numbers():
for i in range(5):
time.sleep(1) # Simulating some work
print(f"Thread: {threading.current_thread().name}, Number: {i}")
# Create a thread
my_thread = threading.Thread(target=print_numbers)
# Start the thread
my_thread.start()
# Main thread continues to execute independently
for i in range(5):
time.sleep(1)
print(f"Main Thread, Number: {i}")
In this example, a thread named my_thread
is created to execute the print_numbers
function. The start()
method initiates the execution of the thread, and both the main thread and my_thread
run concurrently.
2.3 Thread Lifecycle
Understanding the lifecycle of a thread is essential for managing threads effectively. The typical lifecycle includes the following stages:
- Creation: The thread is created.
- Start: The thread transitions from the “created” state to the “running” state.
- Running: The thread is actively executing its task.
- Blocked: The thread is temporarily inactive (e.g., waiting for I/O or synchronization).
- Termination: The thread completes its execution and is terminated.
This section provides an overview of the basics of threading in Python, focusing on thread creation, starting, and the thread lifecycle. In the next sections, we’ll explore advanced concepts, synchronization, and best practices for effective threading in Python.
Thread Synchronization
Thread synchronization is crucial when multiple threads share resources or data to prevent race conditions and ensure data consistency. Python provides synchronization mechanisms such as locks and semaphores to manage thread interactions effectively.
3.1 Importance of Synchronization
In a multithreaded environment, threads may access shared resources concurrently, leading to potential conflicts and data corruption. Synchronization mechanisms help coordinate thread execution to maintain data integrity and prevent unpredictable behavior.
3.2 Locks and Semaphores
3.2.1 Locks
A lock (or mutex) is a synchronization primitive that allows only one thread to access a shared resource at a time. Here’s an example demonstrating the use of a lock:
import threading
# Shared resource
shared_resource = 0
# Create a lock
lock = threading.Lock()
# Function to increment the shared resource
def increment_shared_resource():
global shared_resource
for _ in range(100000):
with lock:
shared_resource += 1
# Create two threads
thread1 = threading.Thread(target=increment_shared_resource)
thread2 = threading.Thread(target=increment_shared_resource)
# Start the threads
thread1.start()
thread2.start()
# Wait for threads to finish
thread1.join()
thread2.join()
print(f"Final value of shared resource: {shared_resource}")
In this example, the with lock:
statement ensures that only one thread can execute the critical section (the block of code inside the with
statement) at a time, preventing race conditions.
3.2.2 Semaphores
A semaphore is a more generalized synchronization primitive that allows multiple threads to access a shared resource simultaneously, up to a specified limit. Here’s a simple example:
import threading
# Shared resource
shared_resource = 0
# Create a semaphore with a limit of 2
semaphore = threading.Semaphore(2)
# Function to increment the shared resource
def increment_shared_resource():
global shared_resource
with semaphore:
# Note: The original example code inside the 'with' block was incorrect for illustrating semaphore limits effectively.
# A typical semaphore use case would involve accessing a limited pool of resources, not just incrementing a counter.
# However, sticking to the original code structure for conversion:
temp_val = shared_resource # Read
time.sleep(0.0001) # Simulate some work inside the critical section
shared_resource = temp_val + 1 # Write
# Creating more threads than the semaphore limit to show its effect
threads = []
for _ in range(5): # Create 5 threads, but semaphore allows only 2 at a time
thread = threading.Thread(target=increment_shared_resource)
threads.append(thread)
thread.start()
# Wait for threads to finish
for thread in threads:
thread.join()
print(f"Final value of shared resource (with Semaphore): {shared_resource}")
# Note: The result might still be incorrect due to the nature of the increment operation
# even with the semaphore if not designed carefully for this specific task.
# A lock is usually more appropriate for simple counter increments.
In this example (adapted slightly for clarity), the semaphore allows up to two threads to access the critical section simultaneously. This can be useful in scenarios where limiting concurrent access to a resource pool is necessary.
3.3 Deadlocks and How to Avoid Them
Deadlocks occur when two or more threads are blocked forever, each waiting for the other to release a lock. Avoiding deadlocks involves careful design and adherence to best practices:
- Lock Ordering: Acquire locks in a consistent order across all threads to prevent circular waiting.
- Lock Timeout: Use a timeout when acquiring locks to avoid indefinite blocking.
Understanding and implementing thread synchronization is crucial for writing robust and reliable multithreaded programs in Python. The use of locks and semaphores helps manage shared resources efficiently and prevents potential issues arising from concurrent access.
Thread Communication
In multithreaded applications, threads often need to communicate and share data. Effective communication between threads is essential for building coherent and synchronized concurrent programs in Python. This section explores various mechanisms for thread communication, such as shared data and inter-thread communication tools like queues and event objects.
4.1 Sharing Data between Threads
Sharing data between threads requires careful synchronization to avoid data corruption or race conditions. Python provides several mechanisms for safe data sharing, such as locks and thread-safe data structures. Here’s an example using a Lock
to safely share data:
import threading
# Shared data
shared_data = 0
# Create a lock for synchronization
data_lock = threading.Lock()
# Function to modify the shared data
def modify_shared_data():
global shared_data
for _ in range(100000):
with data_lock:
shared_data += 1
# Create two threads
thread1 = threading.Thread(target=modify_shared_data)
thread2 = threading.Thread(target=modify_shared_data)
# Start the threads
thread1.start()
thread2.start()
# Wait for threads to finish
thread1.join()
thread2.join()
print(f"Final value of shared data: {shared_data}")
In this example, the with data_lock:
statement ensures that only one thread can modify the shared_data
at a time, preventing data corruption.
4.2 Using Queues for Thread Communication
Queues provide a convenient way for threads to communicate by passing messages or data between them. The queue
module in Python facilitates the implementation of thread-safe queues. Here’s an example:
import threading
import queue
import time
# Create a thread-safe queue
message_queue = queue.Queue()
# Function to produce messages
def produce_messages():
for i in range(5):
time.sleep(1)
msg = f"Message {i}"
print(f"Producing: {msg}")
message_queue.put(msg)
message_queue.put("STOP") # Sentinel value to stop consumer
# Function to consume messages
def consume_messages():
while True:
message = message_queue.get() # Blocks until an item is available
print(f"Consumed: {message}")
if message == "STOP":
break
# Process the message...
message_queue.task_done() # Indicate that the task is done (optional but good practice)
# Create two threads
producer_thread = threading.Thread(target=produce_messages)
consumer_thread = threading.Thread(target=consume_messages)
# Start the threads
producer_thread.start()
consumer_thread.start()
# Wait for the producer to finish producing messages
producer_thread.join()
# Wait for the consumer to process all items (using join on queue or thread)
# message_queue.join() # Waits until task_done() is called for all items
consumer_thread.join() # Waits for the consumer thread to terminate
print("Communication finished.")
In this example, the producer thread produces messages, and the consumer thread consumes them from the queue. The use of a queue ensures that the communication is thread-safe.
4.3 Event Objects for Signaling
Event objects provide a way for one thread to signal another thread that a particular event has occurred. Here’s an example demonstrating the use of an event to signal a thread:
import threading
import time
# Create an event object
event = threading.Event()
# Function to wait for the event
def wait_for_event():
print(f"{threading.current_thread().name}: Waiting for the event...")
event.wait() # Blocks until the event is set
print(f"{threading.current_thread().name}: Event has been set!")
# Function to set the event
def set_event():
time.sleep(2)
print(f"{threading.current_thread().name}: Event is being set!")
event.set() # Sets the event, allowing the waiting thread(s) to proceed
# Create two threads
thread1 = threading.Thread(target=wait_for_event, name="WaiterThread")
thread2 = threading.Thread(target=set_event, name="SetterThread")
# Start the threads
thread1.start()
thread2.start()
# Wait for both threads to finish
thread1.join()
thread2.join()
print("Event signaling finished.")
In this example, thread1
waits for the event to be set using event.wait()
, while thread2
sets the event after a delay using event.set()
. The use of an event allows threads to synchronize and coordinate their activities.
Understanding and effectively using these communication mechanisms is crucial for building robust and efficient multithreaded applications in Python. Whether through shared data, queues, or event objects, thread communication is a key aspect of concurrent programming.
Thread Safety
Thread safety is a critical consideration in multithreaded programming to ensure that shared data and resources are accessed and modified in a way that avoids conflicts and maintains consistency. In Python, several strategies and best practices can be employed to achieve thread safety.
5.1 Understanding Thread Safety
Thread safety refers to the ability of a program or system to function properly and produce predictable results when multiple threads are executing concurrently. Without proper synchronization, concurrent access to shared data can lead to race conditions, where the outcome of operations becomes dependent on the timing or order of thread execution.
5.2 Immutable Objects and Thread Safety
One effective way to achieve thread safety is by using immutable objects. Immutable objects cannot be modified after creation, eliminating the need for locks or synchronization mechanisms when accessed by multiple threads. Examples of immutable objects in Python include tuples, strings, and frozensets.
import threading
# Immutable object (tuple) example
immutable_data = (1, 2, 3)
# Thread-safe operation on immutable data
def process_immutable_data(data):
# Operations on immutable data are inherently thread-safe
# as the data cannot change.
result = sum(data)
print(f"Thread {threading.current_thread().name}: Result: {result}")
# Create two threads
thread1 = threading.Thread(target=process_immutable_data, args=(immutable_data,), name="T1")
thread2 = threading.Thread(target=process_immutable_data, args=(immutable_data,), name="T2")
# Start the threads
thread1.start()
thread2.start()
# Wait for both threads to finish
thread1.join()
thread2.join()
print("Immutable data processing finished.")
In this example, the immutable_data
tuple is shared among multiple threads without the need for explicit synchronization because tuples are immutable.
5.3 Global Interpreter Lock (GIL) in CPython
In CPython (the standard Python implementation), the Global Interpreter Lock (GIL) is a mutex that protects access to Python objects, preventing multiple threads from executing Python bytecode at the same time within a single process. While the GIL simplifies memory management in CPython, it effectively means that only one thread can hold control of the Python interpreter at any given moment.
Impact:
- CPU-bound tasks: Multithreaded Python programs running CPU-bound tasks (e.g., heavy calculations) often do not achieve true parallelism on multi-core processors because of the GIL. Only one thread runs Python bytecode at a time.
- I/O-bound tasks: For I/O-bound tasks (e.g., network requests, disk reads/writes), the GIL is released while the thread waits for the I/O operation to complete. This allows other threads to run, making threading very effective for concurrency in these scenarios.
Developers should be aware of the GIL’s impact on performance and consider alternative concurrency models, such as multiprocessing (which uses separate processes, each with its own GIL) or asyncio, for CPU-bound tasks that need true parallelism.
Example demonstrating a potential race condition with mutable data (where GIL doesn’t prevent it):
import threading
# Global variable (mutable) shared among threads
shared_counter = 0
# Function to increment the shared counter (NOT thread-safe without a lock)
def increment_counter():
global shared_counter
for _ in range(100000):
# This operation (+=) is not atomic. It involves:
# 1. Read current value of shared_counter
# 2. Calculate new value
# 3. Write new value back
# The GIL can be released between these steps, especially around bytecode instructions.
shared_counter += 1
# Create two threads
thread1 = threading.Thread(target=increment_counter)
thread2 = threading.Thread(target=increment_counter)
# Start the threads
thread1.start()
thread2.start()
# Wait for both threads to finish
thread1.join()
thread2.join()
# The result is likely LESS than 200000 due to the race condition
print(f"Final value of shared counter (without lock): {shared_counter}")
In this example, even with the GIL, the shared_counter
increment is not atomic, and the lack of explicit synchronization (like a Lock
) leads to a race condition.
Understanding thread safety is essential for writing reliable and scalable multithreaded programs. While immutable objects provide a simple approach, developers must also use explicit synchronization for mutable shared state and consider the implications of the GIL in CPython, choosing appropriate concurrency models based on application requirements.
Advanced Threading Concepts
Building upon the basics of threading, advanced concepts in Python provide developers with powerful tools for handling more complex scenarios and achieving optimal performance in multithreaded applications.
6.1 Daemon Threads
Daemon threads in Python are threads that run in the background and do not prevent the main program from exiting. The program will exit once all non-daemon threads have completed, even if daemon threads are still running. Daemon threads are useful for tasks like monitoring, logging, or background services that aren’t critical to the program’s main functionality.
import threading
import time
# Function to run as a daemon thread
def daemon_task():
count = 0
while True:
print(f"Daemon thread running... {count}")
count += 1
time.sleep(1)
# Create a daemon thread
daemon_thread = threading.Thread(target=daemon_task, name="MyDaemon")
daemon_thread.daemon = True # Set the thread as daemon BEFORE starting it
# Start the daemon thread
daemon_thread.start()
# Main thread continues execution for a short while
print("Main thread will run for 3 seconds.")
time.sleep(3)
print("Main thread is done. Exiting.")
# Program exits here, terminating the daemon thread abruptly.
In this example, the daemon_thread
continues running in the background. The program exits after the main thread waits for 3 seconds, automatically terminating the daemon thread without needing an explicit join()
.
6.2 ThreadPoolExecutor and Concurrent Futures
The concurrent.futures
module provides a high-level interface for asynchronously executing callables (like functions). The ThreadPoolExecutor
class creates and manages a pool of worker threads, making it easy to submit tasks and manage concurrency without manually creating and joining individual threads.
import concurrent.futures
import time
import random
# Function to simulate a time-consuming task
def time_consuming_task(task_id):
sleep_time = random.uniform(0.5, 2.0)
print(f"Task {task_id}: Started, will sleep for {sleep_time:.2f}s.")
time.sleep(sleep_time)
result = f"Result from Task {task_id}"
print(f"Task {task_id}: Completed.")
return result
# List of task identifiers
task_ids = range(1, 6) # Tasks 1 to 5
# Use ThreadPoolExecutor to parallelize tasks
# max_workers defines the maximum number of threads in the pool
with concurrent.futures.ThreadPoolExecutor(max_workers=3) as executor:
# Submit tasks for execution - returns Future objects
# Method 1: Using submit() and as_completed()
# futures = [executor.submit(time_consuming_task, i) for i in task_ids]
# results = []
# for future in concurrent.futures.as_completed(futures):
# try:
# results.append(future.result())
# except Exception as e:
# print(f"Task generated an exception: {e}")
# Method 2: Using map() (simpler for applying same function to iterable)
# map() returns results in the order tasks were submitted
results = list(executor.map(time_consuming_task, task_ids))
print("\nAll tasks submitted to the pool have completed.")
print("Results:", results)
In this example, tasks are submitted to a ThreadPoolExecutor
with 3 worker threads. The executor.map()
function efficiently applies the task function to each item in task_ids
and collects the results concurrently.
6.3 Multithreading vs. Multiprocessing
Understanding the difference and trade-offs between multithreading and multiprocessing is crucial for choosing the right concurrency model in Python:
-
Multithreading (
threading
,concurrent.futures.ThreadPoolExecutor
):- Uses multiple threads within a single process.
- Threads share the same memory space (easier data sharing, but requires careful synchronization).
- Subject to the GIL in CPython, limiting true parallelism for CPU-bound tasks.
- Excellent for I/O-bound tasks (networking, disk I/O) where threads spend time waiting.
- Lower memory overhead compared to multiprocessing.
-
Multiprocessing (
multiprocessing
,concurrent.futures.ProcessPoolExecutor
):- Uses multiple independent processes.
- Each process has its own memory space and its own Python interpreter (and GIL).
- Achieves true parallelism for CPU-bound tasks on multi-core systems by bypassing the GIL.
- Data sharing between processes requires explicit inter-process communication (IPC) mechanisms (e.g.,
Queue
,Pipe
, shared memory), which adds complexity. - Higher memory overhead as each process duplicates resources.
Example comparing performance for a CPU-bound task:
import concurrent.futures
import time
import math
# Function to simulate a CPU-bound task
def cpu_bound_task(n):
# Perform some calculations
start = time.time()
sum(math.sqrt(i) for i in range(1, n + 1))
duration = time.time() - start
# print(f"CPU-bound Task with n={n} completed in {duration:.4f}s.")
return duration
N = 10_000_000 # A reasonably large number for calculation
NUM_TASKS = 4 # Number of tasks to run
# Using multithreading
start_time = time.time()
with concurrent.futures.ThreadPoolExecutor(max_workers=NUM_TASKS) as executor:
results_threading = list(executor.map(cpu_bound_task, [N] * NUM_TASKS))
threading_time = time.time() - start_time
# Using multiprocessing
start_time = time.time()
with concurrent.futures.ProcessPoolExecutor(max_workers=NUM_TASKS) as executor:
results_multiprocessing = list(executor.map(cpu_bound_task, [N] * NUM_TASKS))
multiprocessing_time = time.time() - start_time
print(f"\n--- CPU-Bound Task Comparison ---")
print(f"Number of tasks: {NUM_TASKS}, N = {N}")
# print("Threading results (durations):", results_threading)
print(f"Total time using Threading: {threading_time:.4f}s")
# print("Multiprocessing results (durations):", results_multiprocessing)
print(f"Total time using Multiprocessing: {multiprocessing_time:.4f}s")
# Expected outcome: Multiprocessing should be significantly faster for this CPU-bound task on a multi-core machine.
These advanced concepts empower developers to design scalable and efficient concurrent programs in Python. Whether working with daemon threads, utilizing thread/process pools, or choosing between multithreading and multiprocessing, these concepts provide the flexibility needed to address diverse concurrency challenges.
Common Pitfalls in Threading and How to Avoid Them
Multithreading introduces complexities that can lead to subtle bugs and performance issues. Understanding common pitfalls is essential for writing robust and efficient multithreaded programs.
8.1 Race Conditions
- Pitfall: Occur when the outcome of a computation depends on the non-deterministic timing of concurrent threads accessing and modifying shared mutable data without proper synchronization. Leads to corrupted data and unpredictable program behavior.
- Avoidance: Use synchronization primitives like
threading.Lock
,threading.RLock
,threading.Semaphore
to protect critical sections where shared mutable data is accessed. Ensure only one thread (or a controlled number for semaphores) can modify the data at a time. Alternatively, use thread-safe data structures likequeue.Queue
or design using immutable objects.
# Example already shown in Thread Safety / Synchronization sections using Lock
import threading
shared_variable = 0
lock = threading.Lock() # Use a lock
def modify_shared_variable_safe():
global shared_variable
for _ in range(100000):
with lock: # Protect the critical section
shared_variable += 1
# (Code to create/start/join threads...)
# print("Final value (safe):", shared_variable) # Should be correct
8.2 Priority Inversion
- Pitfall: A high-priority thread becomes blocked waiting for a resource (e.g., a lock) held by a low-priority thread, which in turn might be preempted by medium-priority threads. This effectively “inverts” the intended priorities.
- Avoidance: Standard Python threading doesn’t offer fine-grained priority control or mechanisms like priority inheritance found in real-time operating systems. The best approach in standard Python is careful design:
- Keep critical sections (code holding locks) as short as possible.
- Avoid blocking operations while holding locks.
- Consider alternative designs if priority is critical (e.g., different concurrency models, or specialized libraries/OS features if available).
8.3 Overhead and Scalability Concerns
- Pitfall: Creating and managing a very large number of threads incurs significant overhead (memory for stacks, context switching costs). Performance can degrade instead of improving beyond a certain point.
- Avoidance: Use thread pools (
concurrent.futures.ThreadPoolExecutor
) to limit the number of active threads to a reasonable number (often related to the number of CPU cores or the nature of I/O tasks). Reuse threads instead of creating new ones for every small task. Profile your application to find the optimal pool size.
# Example using ThreadPoolExecutor already shown in Advanced Concepts
import concurrent.futures
def task(item):
# Some computation or I/O operation
print(f"Processing item {item} in thread {threading.current_thread().name}")
time.sleep(0.1)
items = range(20)
# Using ThreadPoolExecutor to manage threads efficiently
with concurrent.futures.ThreadPoolExecutor(max_workers=5) as executor: # Limit to 5 threads
executor.map(task, items)
8.4 Lack of Thread Safety
- Pitfall: Using non-thread-safe libraries or data structures in a multithreaded context without external locking, or incorrectly assuming a piece of code is thread-safe when it accesses shared mutable state.
- Avoidance:
- Always assume shared mutable state needs synchronization unless proven otherwise.
- Consult library documentation to check for thread safety guarantees.
- Use thread-safe alternatives (e.g.,
queue.Queue
instead of a plain list for inter-thread communication). - Encapsulate shared state within classes and protect access using locks within the class methods.
- Prefer immutable objects where possible.
# Example demonstrating need for locking a shared list
import threading
shared_list = []
list_lock = threading.Lock() # Lock for the list
def modify_shared_list(item):
# Simulate some work before accessing list
time.sleep(random.uniform(0, 0.1))
with list_lock: # Protect access to shared_list
shared_list.append(item)
# Simulate work after accessing list
time.sleep(random.uniform(0, 0.1))
threads = [threading.Thread(target=modify_shared_list, args=(i,)) for i in range(10)]
# (Start and join threads...)
# print("Final shared list (safe):", sorted(shared_list)) # Should contain 0-9
By being aware of these pitfalls and actively employing avoidance strategies, you can build more reliable and performant multithreaded Python applications.
Case Studies: Real-world Examples of Python Threading
Examining real-world case studies can provide valuable insights into how Python threading is applied to address specific challenges.
9.1 Web Scraping with Concurrent Requests
- Challenge: A web scraping task involves fetching data from numerous URLs. Performing these requests sequentially is slow because the program spends most of its time waiting for network responses (I/O-bound).
- Solution: Use threading (
ThreadPoolExecutor
) to send multiple HTTP requests concurrently. While one thread waits for a network response, the GIL is released, allowing other threads to initiate their requests or process responses. This significantly speeds up the overall scraping process.
import concurrent.futures
import requests
import time
# List of URLs to scrape
urls = [
"https://httpbin.org/delay/1", # Simulates 1 second delay
"https://httpbin.org/delay/2", # Simulates 2 second delay
"https://httpbin.org/delay/1",
"https://httpbin.org/delay/3",
"https://httpbin.org/delay/1",
]
def fetch_data(url):
start_time = time.time()
try:
print(f"Fetching {url}...")
response = requests.get(url, timeout=10)
response.raise_for_status() # Raise HTTPError for bad responses (4xx or 5xx)
duration = time.time() - start_time
print(f"Finished {url} in {duration:.2f}s, Status: {response.status_code}")
return url, response.status_code, len(response.content)
except requests.exceptions.RequestException as e:
duration = time.time() - start_time
print(f"Failed {url} in {duration:.2f}s, Error: {e}")
return url, "Error", str(e)
start_total_time = time.time()
# Use ThreadPoolExecutor for concurrent requests
results = []
# Adjust max_workers based on expected network latency and server limits
with concurrent.futures.ThreadPoolExecutor(max_workers=10) as executor:
future_to_url = {executor.submit(fetch_data, url): url for url in urls}
for future in concurrent.futures.as_completed(future_to_url):
results.append(future.result())
end_total_time = time.time()
print(f"\nWeb scraping completed in {end_total_time - start_total_time:.2f}s")
# print("Results:", results)
- Outcome: The total execution time is much closer to the duration of the longest single request (plus some overhead) rather than the sum of all request durations, demonstrating the effectiveness of threading for I/O-bound tasks.
9.2 Parallelizing CPU-bound Tasks with Multiprocessing
(Note: This case study uses multiprocessing, correctly identified in the original text as the better approach for CPU-bound tasks, contrasting with threading)
- Challenge: A computationally intensive task, like applying complex filters to multiple large images or performing heavy numerical simulations, needs to run on a multi-core processor.
- Solution: Use multiprocessing (
ProcessPoolExecutor
) instead of threading. This creates separate processes, each with its own Python interpreter and memory space, bypassing the GIL limitations. Each process can run truly in parallel on a different CPU core, significantly speeding up CPU-bound work.
import concurrent.futures
import time
# Note: Pillow (PIL fork) and requests would need to be installed: pip install Pillow requests
# This example structure assumes image processing functions exist
from PIL import Image, ImageFilter
from io import BytesIO
import requests # Used to get images, which is I/O, but the focus is the CPU-bound part
# List of image URLs to download and process
# Replace with actual valid image URLs for testing
image_urls = [
"https://via.placeholder.com/300.png/09f/fff",
"https://via.placeholder.com/400.png/f90/fff",
"https://via.placeholder.com/350.png/90f/fff",
"https://via.placeholder.com/200.png/0f9/fff",
]
def download_image(url):
# I/O bound part
try:
response = requests.get(url, timeout=10)
response.raise_for_status()
return Image.open(BytesIO(response.content))
except Exception as e:
print(f"Failed to download {url}: {e}")
return None
def apply_cpu_intensive_filter(image):
# CPU-bound part - Simulate with multiple filters
if image is None: return None
start_time = time.time()
processed_image = image.filter(ImageFilter.GaussianBlur(radius=5))
processed_image = processed_image.filter(ImageFilter.CONTOUR)
# Add more complex operations here...
# time.sleep(0.5) # Simulate more work if needed
duration = time.time() - start_time
print(f"Applied filter in {duration:.2f}s")
return processed_image
def process_image(image_url):
print(f"Processing {image_url}...")
original_image = download_image(image_url)
processed_image = apply_cpu_intensive_filter(original_image)
if processed_image:
print(f"Finished processing {image_url} with size {processed_image.size}")
# processed_image.save(f"processed_{image_url.split('/')[-1]}.png") # Optional save
return image_url, "Success"
else:
return image_url, "Failed"
start_total_time = time.time()
# Using ProcessPoolExecutor for parallel CPU-bound processing
results = []
# max_workers usually defaults to number of CPU cores
with concurrent.futures.ProcessPoolExecutor() as executor:
future_to_url = {executor.submit(process_image, url): url for url in image_urls}
for future in concurrent.futures.as_completed(future_to_url):
results.append(future.result())
end_total_time = time.time()
print(f"\nImage processing completed in {end_total_time - start_total_time:.2f}s")
# print("Results:", results)
- Outcome: Multiprocessing significantly reduces the total time for CPU-bound tasks compared to sequential execution or using threading (which would be limited by the GIL). The speedup approaches the number of CPU cores available for truly parallelizable tasks.
These case studies highlight the importance of choosing the right concurrency model (threading for I/O, multiprocessing for CPU) based on the nature of the task to achieve the best performance improvements in Python.
Conclusion
In conclusion, Python threading is a powerful feature that allows developers to create concurrent and efficient programs. Threading becomes especially relevant when dealing with tasks that can be performed concurrently, such as I/O-bound operations, parallelizable computations (within GIL limits), and asynchronous tasks. However, it’s essential to be aware of potential challenges and best practices to ensure the reliability and performance of multithreaded applications.
Key Takeaways:
- Concurrency vs. Parallelism: Python threading enables concurrency (managing multiple tasks over time). Due to Python’s Global Interpreter Lock (GIL) in CPython, true parallelism (simultaneous execution on multiple cores) for CPU-bound tasks is limited with threading; multiprocessing is often needed for that. Threading remains highly effective for I/O-bound and asynchronous operations.
- Synchronization: Careful synchronization is necessary when threads share mutable data to prevent race conditions and ensure thread safety. Mechanisms like Locks, Semaphores, and thread-safe data structures (
queue.Queue
) are crucial for managing shared resources. - Advanced Concepts: Daemon threads,
ThreadPoolExecutor
, andconcurrent.futures
offer high-level abstractions for managing threads efficiently, simplifying development and improving resource management. - Multiprocessing vs. Multithreading: The choice depends fundamentally on the task type: multithreading excels for I/O-bound tasks, while multiprocessing is generally required for CPU-bound tasks needing true parallelism.
- Common Pitfalls: Be aware of race conditions, deadlocks, priority inversion (less common/manageable in standard Python), and scalability concerns. Mitigate these through proper synchronization, careful design, and using abstractions like thread pools.
- Case Studies: Real-world examples like concurrent web scraping (I/O-bound, good for threads) and parallel image processing (CPU-bound, better with multiprocessing) demonstrate the practical application and benefits of choosing the appropriate model.
In summary, Python threading is a valuable tool for concurrent programming, offering flexibility and performance improvements when used appropriately for the right kinds of tasks. By understanding the nuances of threading, applying synchronization techniques diligently, being mindful of the GIL, and leveraging advanced concepts and tools, developers can harness the power of concurrency in Python to build responsive, efficient, and scalable applications.
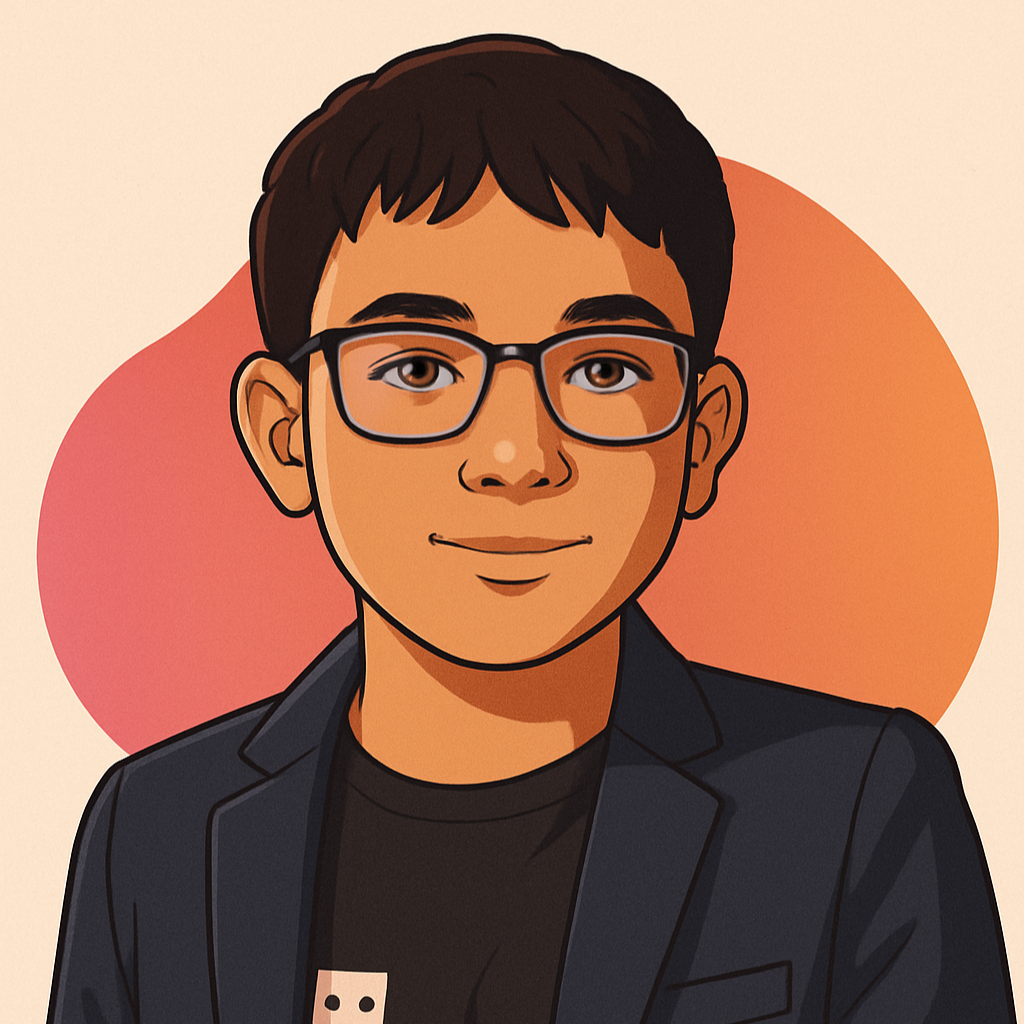
Solo Developer | Python & JS | FastAPI, Django, Next.js, Svelte